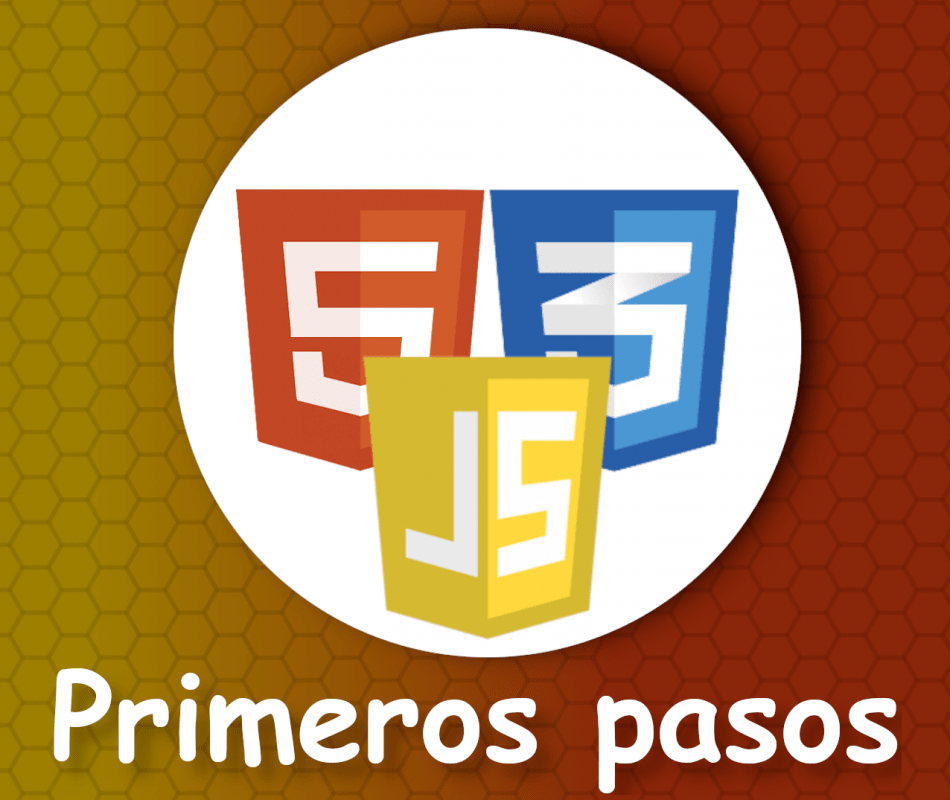
The if is the control structure par excellence that allows us to make decisions according to a condition to be evaluated; Its definition looks like the following:
if(condition) {
...
}
If the condition is met, that is, its value is true, then everything we put between the braces will be executed; which can be anything, any instruction we have seen up to this point and many more; we can even place another condition, that is, another conditional or if; for example:
var bool = true;
n=0
if(bool) {
n = n + 5
}
n
But what if we bool is not true:
var bool = false;
n=0
if(bool) {
n = n + 5
}
n
Control structures: if-else
Now, what happens if we also want to determine "if this is true then do this" or "if this is not true, do this"; that is, create a flow whether the condition is correct or not; In these types of situations we can perfectly use other types of structures known as the if-else
n=0
if(bool) {
n = n + 5
} else {
n = 2
}
n
age = 21
olderAge = false
if(age >= 18) {
olderAge = true
} else {
olderAge = false
}
Older
Control structures: nested if
We can chain the conditional structure to ask for several conditions; we can create as many nestings as we want; As you can see in this example we have a nest to ask to find out if the person is a minor; as you can see we have two conditions in a single conditional structure that would be the entire body that you can see.
In short, to create a nested if or nested conditional, we have to use the else if structure and we can create as many of these structures as we want, as we need.
status = ""
age = 12
if(age < 12) {
status = "You are a boy"
} else if(age < 18) {
status = "You are a teenager"
} else {
status = "You are an adult"
}
status = ""
age = 12
if(age < 12) {
status = "You are a boy"
} else if(age < 18) {
status = "You are a teenager"
} else if(age >= 18) {
status = "You are an adult"
} else if(age >= 60) {
status = "Elderly"
}
I agree to receive announcements of interest about this Blog.
The if is the control structure par excellence that allows us to make decisions according to a condition to be evaluated.
- Andrés Cruz