In this post we will explain how to create a vertical carousel that occupies the entire screen (fullscreen); whose movement is carried out using the mouse wheel or through a simple left side menu with the characteristic vignettes located on the left side of the carousel. In general, this type of carousel is widely used in modern systems since they are very visual and allow you to present a gallery of images in a very practical way.
Defining the HTML structure of the vertical gallery
First we will create the HTML structure of our document, it will be comprised of a fixed side navigation menu:
<nav>
<li class="active"><a href="#polyscape-1"></a></li>
<li><a href="#polyscape-2"></a></li>
<li><a href="#polyscape-3"></a></li>
<li><a href="#polyscape-4"></a></li>
<li><a href="#polyscape-5"></a></li>
</nav>
And each of the backgrounds that will occupy the entire screen, with the left side menu defined above, is used to move between each of the backgrounds that we define below:
<div id="polyscape-1" class="polyscape-background"></div>
<div id="polyscape-2" class="polyscape-background"></div>
<div id="polyscape-3" class="polyscape-background"></div>
<div id="polyscape-4" class="polyscape-background"></div>
<div id="polyscape-5" class="polyscape-background"></div>
An important point is to note that in the menu we define in the href attribute the identifier (id attribute) of the funds, which means that we use the destination of these links to represent the start of these containers due to the basic operation that the HTML API allows .
CSS for sticky menu and fullscreen gallery
The following CSS is used to set the side menu on the left side and place it in the center, for this properties such as position, display, justify-content, etc. are used:
nav {
position: fixed;
left: 40px;
top: 0;
height: 100%;
list-style: none;
display: flex;
flex-direction: column;
justify-content: center;
z-index: 10;
}
nav li {
padding: 10px 0;
}
nav li a {
position: relative;
display: block;
}
nav li a:after {
content: "";
display: block;
width: 16px;
height: 16px;
border-radius: 50%;
border: 2px solid #FFF;
transition: 0.2s;
}
nav li.active a:after {
background: #FFF;
}
nav.dark li a:after {
border: 2px solid #444;
}
nav.dark li.active a:after {
background: #444;
}
Now we define the CSS so that the backgrounds occupy 100% of the screen; for this, the CSS defined in polyscape-background is mainly used:
.polyscape-background {
width: 100vw;
height: 100vh;
overflow: hidden;
position: relative;
display: flex;
justify-content: center;
align-items: center;
background-attachment: fixed;
background-position: center;
background-size: cover;
}
.polyscape-background > div {
position: absolute;
}
#polyscape-1 {
background-image: url(../images/polyscape-1/mountain.jpg) ;
}
#polyscape-2 {
background-image: url(../images/polyscape-2/mist.jpg) ;
}
#polyscape-3 {
background-image: url(../images/polyscape-3/space.jpg) ;
}
#polyscape-4 {
background-image: url(../images/polyscape-4/lake.jpg) ;
filter: grayscale(25%);
}
#polyscape-5 {
background-image: url(../images/polyscape-5/mountain.jpg) ;
}
How do we activate/deactivate bullets in the menu
To do this we use the following jQuery code:
This code captures the side menu click events and overrides the default behavior and programmatically scrolls the background via an animation:
menuItems.click(function(e){
var href = $(this).attr("href"),
offsetTop = href === "#" ? 0 : $(href).offset().top;
$('html, body').stop().animate({
scrollTop: offsetTop
}, 300);
e.preventDefault();
});
This code records in an array the height of each of the containers that contains the fullscreen background:
scrollItems = menuItems.map(function(){
var item = $($(this).attr("href"));
if (item.length) { return item; }
});
Finally, the following code determines the user's position with respect to the backgrounds to activate/deactivate the side menu bullets:
// Bind to scroll
$(window).scroll(function(){
// Get container scroll position
var fromTop = $(this).scrollTop();
// Get id of current scroll item
var cur = scrollItems.map(function(){
if ($(this).offset().top-100 < fromTop)
return this;
});
// Get the id of the current element
cur = cur[cur.length-1];
var id = cur && cur.length ? cur[0].id : "";
if (lastId !== id) {
lastId = id;
// Set/remove active class
if (id=='polyscape-5') {
menu.addClass('dark');
}
else {
menu.removeClass('dark');
}
menuItems
.parent().removeClass("active")
.end().filter("[href='#"+id+"']").parent().addClass("active");
}
});
You can consult the original code of the experiment at the following link:5 Beautiful Image Effects With CSS Shapes and Filters.
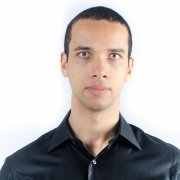
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter