Este material forma parte de mi curso y libro completo; puedes adquirirlos desde el apartado de libros y/o cursos.
In this article we will see how to increase or reduce the contrast of an image with HTML5; We will also talk a little about what the process would be like to increase the brightness of an image; which is a similar case.
These are some of the methods, technologies and concepts that will be used to carry out the development of this exercise:
- Canvas: To use it as a canvas and draw an image.
- getImageData(): Allows you to obtain data about the image drawn on the Canvas (ImageData):
- ImageData.width: Width in pixels of the ImageData.
- ImageData.height: Height in pixels of the ImageData.
- ImageData.resolution: The pixel density of the image.
- ImageData.data: One-dimensional array containing the data in RGBA (in that order), with integers between 0-255; represents the colors that make up the image drawn on the Canvas; in other
- words; the values of the pixels that make up the image drawn on the Canvas.
- img.onload: Event that appears once the image has been loaded.
The contrast formula
It is beyond the scope of this article to define contrast to its full extent; However, we can define contrast as a variation of intensities that allows increasing the luminosity between the dark and light areas of an image, allowing better focus and clarity of the image; the formula used at the pixel level will be the following:
valorNuevo = ( valorAnterior - 128) * tan(ángulo) + 128
Where:
- previousvalue: It is the original value of a pixel in the image.
- angle: We calculate the angle as: Math.tan(val * Math.PI / 180.0);
- val: The value of the range type field managed by the user.
You can get more information about the formula at the following link: Generation Imagenes.
Defining the HTML
First we will need a Canvas; which we will use as a canvas to paint an image and be able to manipulate it at the pixel level:
<canvas id="canvas">
<p>Tu navegador no soporta Canvas.</p>
</canvas>
A range field (type="range") to easily increase and decrease contrast:
<input type="range" id="contrast" min="-90" max="90" step="5" value="0">
Defining JavaScript
Global variables:
var canvas = document.getElementById('canvas');// canvas
var ctx = canvas.getContext('2d'); // contexto
var contrast = document.getElementById("contrast");// input de tipo rango
var srcImg = "image.png";// imagen fuente
First it is necessary to create an object of type image to which a source image is established that will be used to paint it on the Canvas with the drawImage() method:
// nueva imagen
img = new Image();
img.src = srcImg;
img.onload = function() {
// reescalamos el canvas a las dimenciones de la imagen
canvas.width = img.width;
canvas.height = img.height;
// dibujamos la imagen en el Canvas
ctx.drawImage(this, 0, 0);
};
The following function allows you to increase the contrast of the image painted on the Canvas:
// aumenta el contraste
function AddContrast(val) {
//combino la formula para obtener el contraste con el valor obtenido del elemento ranges
var contrast = Math.tan(val * Math.PI / 180.0);
// reescalamos el canvas a las dimenciones de la imagen
canvas.width = img.width;
canvas.height = img.height;
// dibujamos la imagen en el Canvas
ctx.drawImage(img, 0, 0);
// obtenemos el ImageData
var imgd = ctx.getImageData(0, 0, canvas.width, canvas.height);
var pix = imgd.data;
// cambiamos el contraste
for (var i = 0, n = pix.length; i < n; i += 4) {
//incremento los valores rojo, verde y azul de cada pixel
pix[i] = rangeColor(128 + (pix[i] - 128) * contrast);
pix[i + 1] = rangeColor(128 + (pix[i + 1] - 128) * contrast);
pix[i + 2] = rangeColor(128 + (pix[i + 2] - 128) * contrast);
}
// retornamos la data modificada al Canvas
ctx.putImageData(imgd, 0, 0);
}
Analyzing the previous function...
We create an object of type Image and assign it a source image.
img = new Image();
img.src = srcImg;
We obtain the imageData from the Canvas and operate at the pixel level with the ImageData.data:
// obtenemos el ImageData var imgd = ctx.getImageData(0, 0, canvas.width, canvas.height); var pix = imgd.data;
Finally we alter the pixel values by adding contrast to the image to the pixels in its RGB notation:
for (var i = 0, n = pix.length; i < n; i += 4) {
//incremento los valores rojo, verde y azul de cada pixel
pix[i] = rangeColor(128 + (pix[i] - 128) * contrast);
pix[i + 1] = rangeColor(128 + (pix[i + 1] - 128) * contrast);
pix[i + 2] = rangeColor(128 + (pix[i + 2] - 128) * contrast);
}
To ensure that the color does not go out of the range 0-255 we use the following function:
// valida que el color este en un rango valido function rangeColor(pix) { if (pix < 0) pix = 0; if (pix > 255) pix = 255; return pix; }
The bright
As for brightness, it consists of adding a constant (K) that is in the range 0-255; so just by modifying this section of code:
for (var i = 0, n = pix.length; i < n; i += 4) {
//incremento los valores rojo, verde y azul de cada pixel
pix[i] = rangeColor(128 + (pix[i] - 128) * contrast);
pix[i + 1] = rangeColor(128 + (pix[i + 1] - 128) * contrast);
pix[i + 2] = rangeColor(128 + (pix[i + 2] - 128) * contrast);
}
For this other:
for (var i = 0, n = pix.length; i < n; i += 4) {
//cambio los valores rojo, verde y azul de cada pixel
pix[i] = rangeColor(pix[i] + K);
pix[i + 1] = rangeColor(pix[i + 1] + K);
pix[i + 2] = rangeColor(pix[i + 2] + K);
}
You can easily change the brightness of an image with just HTML5; The constant K can be obtained from a range type field as in the case of the contrast:
<input type="range" id="brightness" min="0" max="255" step="1" value="0">
Links of interest
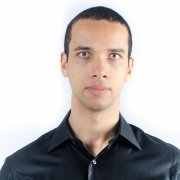
Desarrollo con Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter