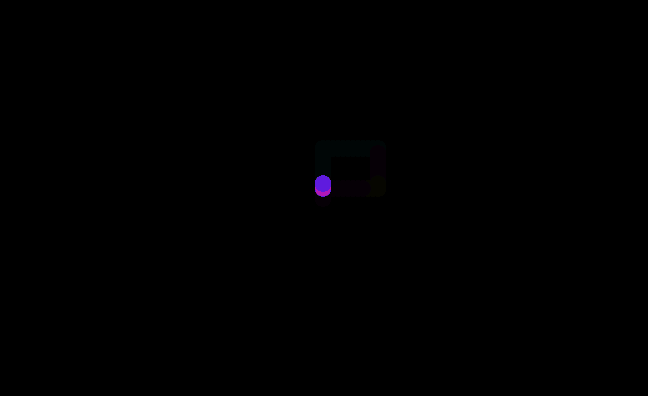
So far we have seen several experiments using Canvas and JavaScript in which we perform various animations, but these do not depend on any other agent to vary their behavior:
- ¿CÓMO CREAR UN EFECTO DE ONDA CON CANVAS Y JAVASCRIPT?
- ¿CÓMO CREAR UN PUNTO LUMINOSO CON JAVASCRIPT Y CANVAS?
- CREANDO PARTÍCULAS CON JAVASCRIPT Y CANVAS
- CÓMO CREAR ANILLOS DE CIRCULOS EN JAVASCRIPT Y CANVAS
In this entry we will see a small experiment where we interact with the Canvas through keyboard events, specifically we will use the keyboard direction arrows.
Making animations with JavaScript and Canvas which depend on external agents such as keyboard or mouse are very simple.
As you can imagine, when introducing the concept of animation, the use of the requestAnimationFrame() function is being hinted at, which allows animations to be carried out with the Canvas in an efficient way; although you must take into consideration compatibility with old browsers as it is part of the HTML5 API; You can get more information at: EL SECRETO DE LAS ANIMACIONES EN JAVASCRIPT.
Starting the experiment with Canvas, animations and keyboard events (JavaScript)
The experiment consists of moving a small circle throughout the Canvas, leaving a slight trail in its wake using the direction arrows on the keyboard.
Initializing the keyboard event
We will associate the event to the document although you can also associate it to the Canvas if you prefer:
document.addEventListener('keydown', function(e) { }, false);
In the 'keydown' listener we will associate the code of the keys with which we want the Canvas to interact and we will update variables that are taken when redrawing the circle using the requestAnimationFrame() function.
You can search for the codes associated with pressing the arrow keys or simply place an alert or console.log and consult the code when you press the keyboard.
Once we get the codes we can form the following conditionals to increase/decrease a series of variables:
document.addEventListener('keydown', function (e) {
lastDownTarget = event.target;
if (e.keyCode === 37) {
x-=v;
}
if (e.keyCode === 38) {
y-=v;
}
if (e.keyCode === 39) {
x+=v;
}
if (e.keyCode === 40) {
y+=v;
}
}, false);
- The variables x and y are global and represent the position of the circle at a given moment; By default, both are initialized to zero.
- The variable v is a global and constant value that expresses the speed of the circle when moving.
x represents the position of the circle on the X axis and y represents the position of the circle on the Y axis.
Painting on the Canvas
So far we have seen how to update a couple of variables using keyboard events; these variables will be used to draw the circle in a certain position, now we will see how to draw the circle using the positions:
function draw() {
$.fillStyle = 'hsla(' + (x*y)/100 + ',100%, 50%, 1)';
$.beginPath();
$.arc(x, y, 8, 0, dosPi);
$.fill();
}
Very simple, we simply consult the global variables x and y that we update in another function.
In other words; When updating the variables through keyboard events, they must also be updated when repainting the Canvas through the requestAnimationFrame() function:
function go() {
$.fillStyle = 'hsla(0,0%,0%,.08)';
$.fillRect(0, 0, w, h);
if (x <= 0)
x = 0;
if (y <= 0)
y = 0;
if (x >= w)
x = w;
if (y >= h)
y = h;
draw();
window.requestAnimationFrame(go);
}
The complete code of the Canvas experiment:
var c = document.getElementById('canv');
var w = c.width = window.innerWidth;
var h = c.height = window.innerHeight;
var $ = c.getContext('2d');
var x = w/2;
var y = h/2;
var dosPi = Math.PI * 2;
var v = 5;
window.addEventListener('resize', function () {
c.width = window.innerWidth;
c.height = window.innerHeight;
}, false);
function draw() {
$.fillStyle = 'hsla(' + (x*y)/100 + ',100%, 50%, 1)';
$.beginPath();
$.arc(x, y, 8, 0, dosPi);
$.fill();
}
function go() {
$.fillStyle = 'hsla(0,0%,0%,.08)';
$.fillRect(0, 0, w, h);
if (x <= 0)
x = 0;
if (y <= 0)
y = 0;
if (x >= w)
x = w;
if (y >= h)
y = h;
draw();
window.requestAnimationFrame(go);
}
go();
document.addEventListener('keydown', function (e) {
lastDownTarget = event.target;
if (e.keyCode === 37) {
x-=v;
}
if (e.keyCode === 38) {
y-=v;
}
if (e.keyCode === 39) {
x+=v;
}
if (e.keyCode === 40) {
y+=v;
}
}, false);
And in order not to make this entry extremely long, we will leave it here; In later posts we will see how to interact a little more with the Canvas through keyboard events.
You can try the experiment at the following link:
I agree to receive announcements of interest about this Blog.
In this entry we will see a small experiment where with Canvas, through keyboard events, we will specifically use the keyboard direction arrows.
- Andrés Cruz