Todo sobre el widget Scaffold en Flutter
- Andrés Cruz
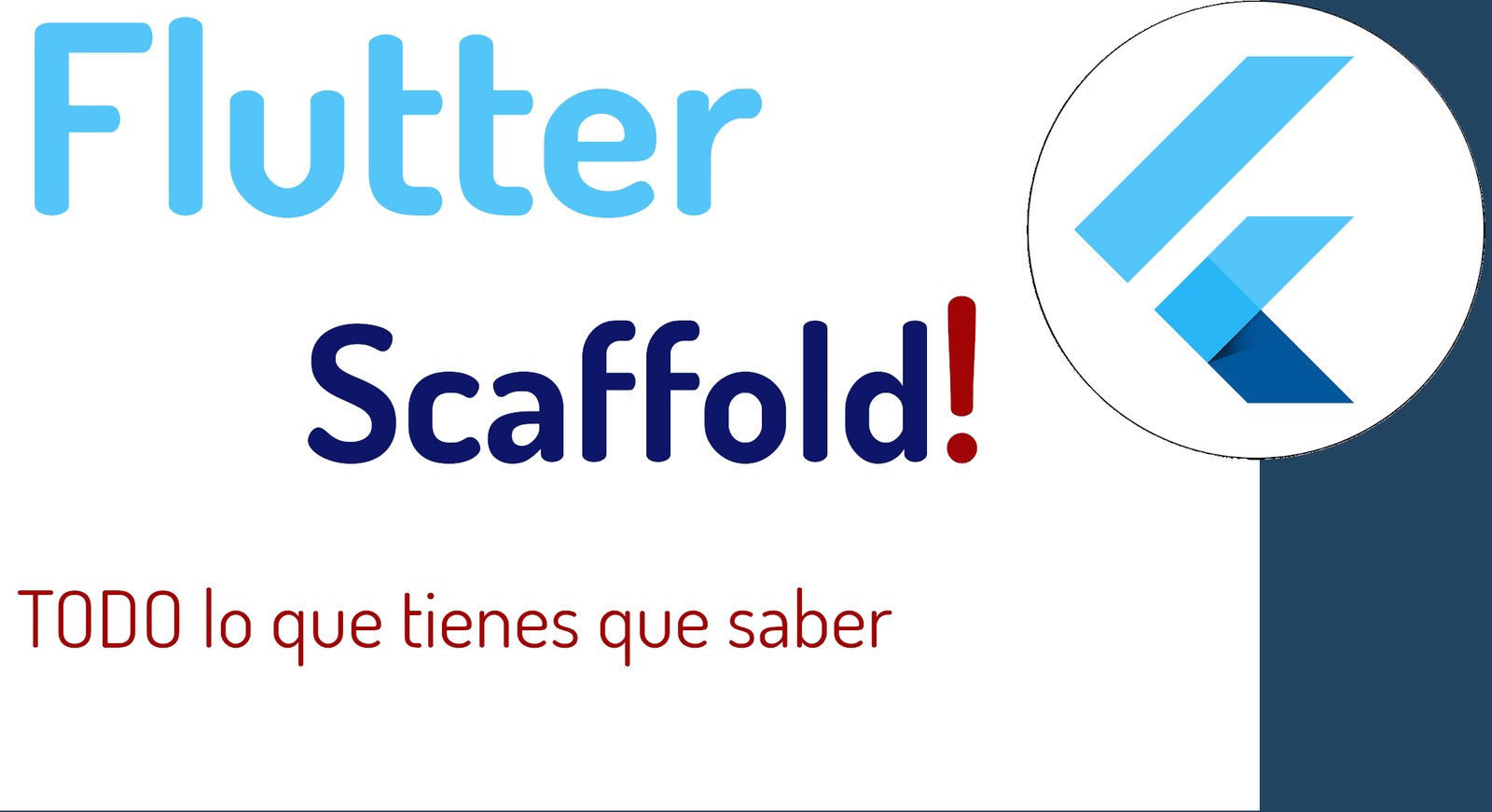
El Scaffold, es un widget hijo del MaterialApp que es donde comienza todo y nos permite emplear elementos/widgets del Material Design, el Scaffold es nuestro pilar en el cual podemos crear más opciones como el botón flotante, el appbar, drawer y mucho más.
Introduciendo esto, una app en Flutter, cuando la creamos, comúnmente luce:
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
Así es como luce nuestra app, con elementos básicos como:
- appBar
- body
- floatingActionButton
Pero estos son solo algunos de los atributos, este widget también contiene algunos atributos adicionales. Veamos la declaración del constructor con parámetros nombrados para aprender sobre ellos:
Scaffold({
Key? key,
PreferredSizeWidget? appBar,
Widget? body,
Widget? floatingActionButton,
FloatingActionButtonLocation? floatingActionButtonLocation,
FloatingActionButtonAnimator? floatingActionButtonAnimator,
List<Widget>? persistentFooterButtons,
Widget? drawer,
void Function(bool)? onDrawerChanged,
Widget? endDrawer,
void Function(bool)? onEndDrawerChanged,
Widget? bottomNavigationBar,
Widget? bottomSheet,
Color? backgroundColor,
bool? resizeToAvoidBottomInset,
bool primary = true,
DragStartBehavior drawerDragStartBehavior = DragStartBehavior.start,
bool extendBody = false,
bool extendBodyBehindAppBar = false,
Color? drawerScrimColor,
double? drawerEdgeDragWidth,
bool drawerEnableOpenDragGesture = true,
bool endDrawerEnableOpenDragGesture = true,
String? restorationId,
})
Hay un montón de atributos que puedes usar, pero ninguno de ellos es obligatorio para usar, aún así, todos los widgets de Scaffold usan el atributo a body para representar el cuerpo de scaffold. Todos estos widgets se explican por sí mismos y la declaración anterior dice lo que esperan. Ahora cambiemos el Scaffold de nuestra aplicación predeterminada:
@override
Widget build(BuildContext context) {
return Scaffold(
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(
Icons.home,
),
label: 'Home',
tooltip: 'Home',
),
BottomNavigationBarItem(
icon: Icon(
Icons.person,
),
label: 'Profile',
tooltip: 'Profile',
),
],
),
drawer: const Drawer(
child: Center(
child: Text(
"Start Drawer",
),
),
),
endDrawer: const Drawer(
child: Center(
child: Text(
"End Drawer",
),
),
),
backgroundColor: Colors.grey[200],
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
El código anterior crea un menú de navegación inferior, un cajón en la posición inicial y final de la pantalla, una barra de navegación inferior y reemplaza la posición del botón de acción flotante en la parte inferior central y se superpone a la barra de navegación inferior. Se parece a esto.
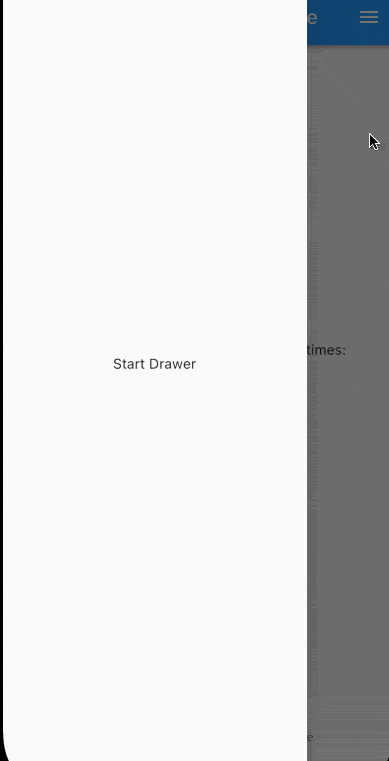
Lectura recomendada:
https://medium.com/@omlondhe/all-about-scaffold-widget-in-flutter-98e674995a43
Acepto recibir anuncios de interes sobre este Blog.
El Scaffold nos permite atar botones de tipo flotante, drawer entre otros más; contiene algunos atributos adicionales. veremos la declaración del constructor con parámetros para saber exactamente como funciona.
- Andrés Cruz