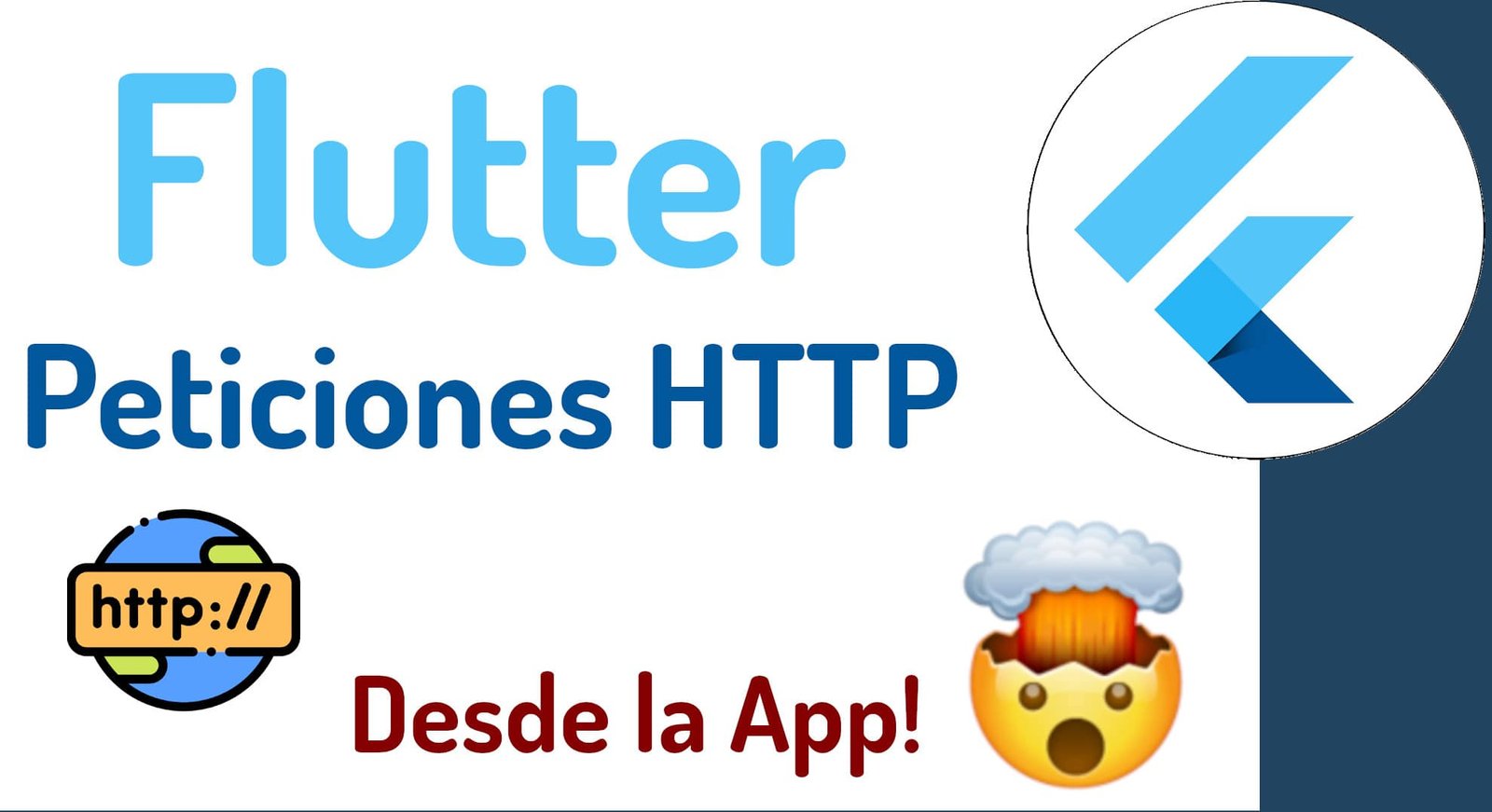
In any application today, it is necessary to be able to communicate with the Internet in order to obtain data, Flutter is no exception, whether you want to create web or mobile applications with it, you must know how to make this type of connection or integration, in this tutorial, we will address this topic.
In this article, you'll create a sample Flutter app that uses the http package to make HTTP requests.
HTTP (Hyper Text Transfer Protocol) is used for effective communication between the "Client" and the "Server" and the message that a client sends to a server is known as an "HTTP request" and that if you have taken my courses, you already You must know since we always make HTTP type connections to intercommunicate systems and Flutter is no exception.
Flutter supports features like HTTP request of all kinds to interface web systems built in Laravel, CodeIgniter... Django, Flash... or anything that uses HTTP requests with Flutter which is period.
Step 1: The first thing is to add the http package to the pubspec.yaml file and run pub get:
https://pub.dev/packages/http
This package allows us to easily make HTTP requests to any other application, including our own.
step 2: import the http package:
import 'package:http/http.dart' as http;
We put the alias of as http so that we can reference all the methods that this package offers us through http.
Step 3: Create a function to send a get request:
Here you can see some code that is pretty self-explanatory: the first thing we need is to define the connection String by means of a Uri, which we pass from a String to a uri.
The following is to make the request, which can be of any type, and in this case it is a request of type post to the previous route and we pass some parameters as an example: as you can suppose, since it is a request to the internet, this takes time some time that we DO NOT know how to determine and that is why we place an await.
Finally we have the answer and we can ask for different data such as status, headers and of course the body that brings us the response data.
var url = Uri.parse('tu-sitio');
var response = await http.post(url, body: {'name': 'doodle', 'color': 'blue'});
print('Response status: ${response.statusCode}');
print('Response body: ${response.body}');
print(await http.read('https://example.com/foobar.txt'));
Explanation of the above function
- http.response (used to request the URL data and store the response)
- Now store the response in the response variable response = await http.get(Uri.parse("your-site"));
- We are parsing the URL here because the URL consists of raw data and we cannot use it.
- Now we need to decode or parse the json using json.decode(response.body) taken from dart:convert package
- In the previous step we decoded the body because the raw data is present in the response body.
more methods - Of course we can make different types of connection with methods of type get, post, put, patch, delete...
Some examples that may interest you and that are part of my Flutter course.
In this first example we see how to make PUT requests to update content by passing an identifier parameter through the URL; In addition to passing through the body a field called "products" which is nothing more than a list of identifiers, we also see how to use the headers, in this case, to pass the authentication token:
final res = await http.put(
Uri.parse("http://10.0.2.2:1337/favorites/${user.favoriteId}"),
body: {
"products": json.encode(productsFavoriteId),
},
headers: {
"Authorization": "Bearer ${user.jwt}"
});
In this second example, we see how to make a GET request to a domain, passing an identifier and protection through an authentication token; then, we evaluate the response to see if it was successful through a 200 request:
final res = await http.get(
Uri.parse("http://10.0.2.2:1337/favorites/${user.favoriteId}"),
headers: {"Authorization": "Bearer ${user.jwt}"});
print(res.statusCode);
if (res.statusCode == 200) {
final resData = json.decode(res.body);
}
In this second example, we see how to send a post request with two fields supplied in the body:
final res = await http.get(Uri.parse("http://10.0.2.2:1337/products"));
final List<dynamic> resData = json.decode(res.body);
final res = await http.post(Uri.parse('http://10.0.2.2:1337/auth/local'), body: {
"identifier": _emailController.text,
"password": _passwordController.text,
});
In all cases, it is important to remember that, they are asynchronous requests, any type of request that is not part of the application, if not of external means, such as accessing the disk, a memory or in this case, an HTTP request, are made through asynchronous processes.
I agree to receive announcements of interest about this Blog.
Sending HTTP requests from an app in Flutter is possible, NECESSARY and very easy with a package; it is fundamental to interconnect applications; Learn how!
- Andrés Cruz