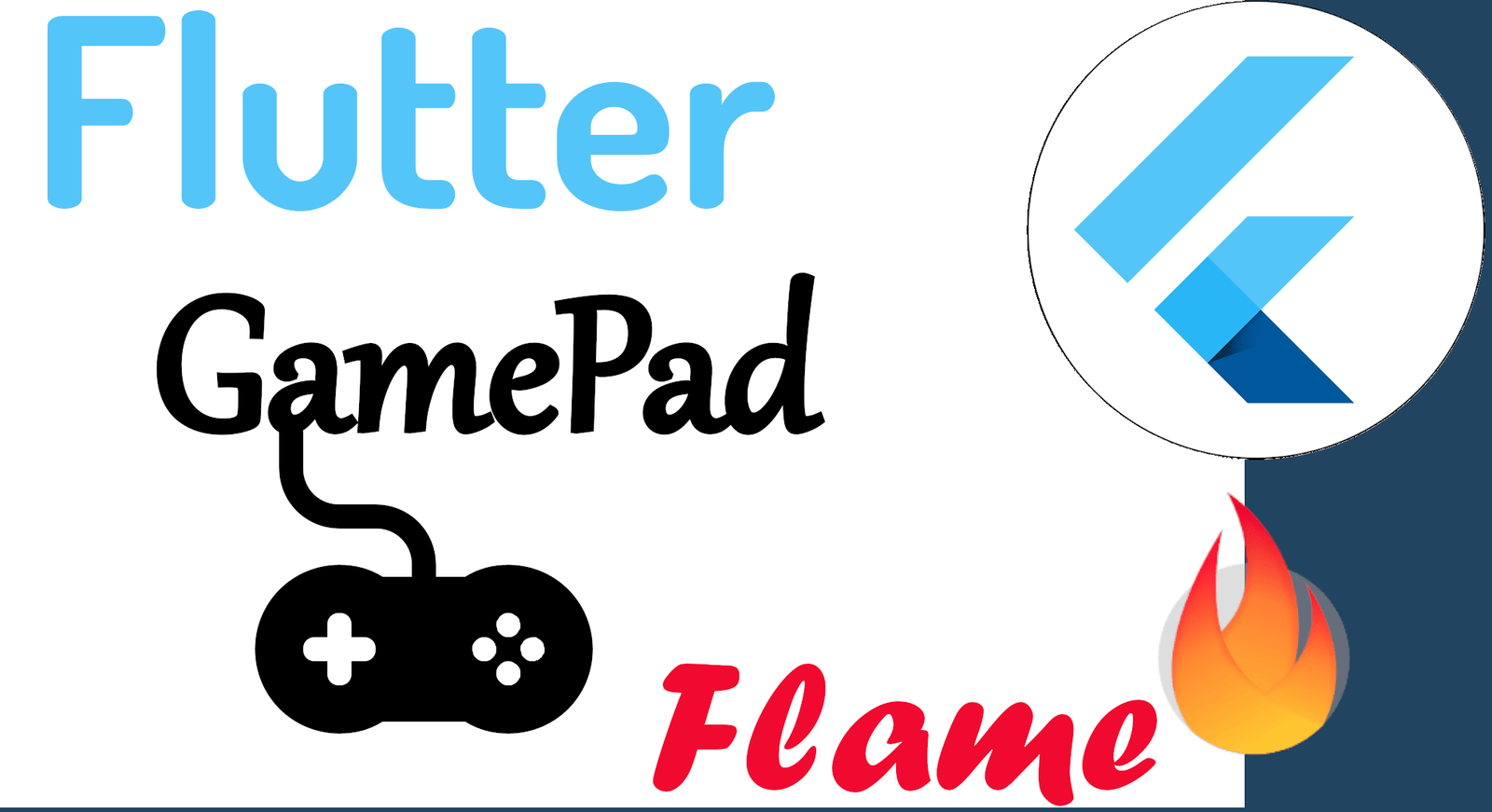
The GamePad is the input device used to interact with a video game and one of the first elements that come to mind when we hear the word "video game" or "video game console"; using these types of controls for our games is not impossible; however, in Flame, we don't have many options; in the official documentation, we will see that at the time these words were written, there is a very short section that mentions the use of the gamepad in Flame:
https://docs.flame-engine.org/latest/flame/inputs/other_inputs.html
The problem with the package that the Flame team mentions:
https://github.com/flame-engine/flame_gamepad
It is that at the time these words were written, it has not been updated for more than 3 years and in software development, which includes video game development, it is a considerably long time, therefore, I personally would not recommend its use since there may be package version problems with the current project, which would cause problems in the implementation.
In Flutter (not directly Flame, but the framework known as Flutter) there are a few plugins that we can use; for example this:
https://pub.dev/packages/gamepads
At the time these words were written, the package is under development, therefore, its documentation is very scarce and its implementation is not entirely user friendly, as we will see in the example section; however, it is a package that has potential and is in constant development, therefore it is the one that we are going to use; It is important to mention that this package is not designed for Flame, like the Sharedpreferences package, but we can use it without problems in a project with Flutter and Flame; currently this pack is not available for web or mobile.
First steps with the gamepads plugin
Let's start by adding the dependency for the above package:
pubspec.yaml
dependencies:
flutter:
sdk: flutter
***
gamepads:
Which has 2 main classes:
- GamepadController: Represents a single currently connected gamepad.
- GamepadEvent: It is an event that is built when an input from a gamepad occurs, in other words, when a key is pressed, an event of this type is built.
The GamepadEvent class contains the following properties:
class GamepadEvent {
// The id of the gamepad controller that fired the event.
final String gamepadId;
// The timestamp in which the event was fired, in milliseconds since epoch.
final int timestamp;
// The [KeyType] of the key that was triggered.
final KeyType type;
// A platform-dependant identifier for the key that was triggered.
final String key;
// The current value of the key.
final double value;
}
The most important would be the key, which indicates the key pressed, and the value, with which, depending on the type of button pressed, we can know the angle or if the button was pressed or released.
The input type can be of two types that is represented by an enumerated type called KeyType:
- analog: Analog inputs have a range of possible values depending on how far/hard they are pressed (they represent analog sticks, back triggers, some kinds of d-pads, etc.)
- button: Buttons have only two states, pressed (1.0) or not (0.0).
Define a listener to detect keys pressed
For this plugin, we have two main uses, knowing the number of connected controls; for it:
gamepads = await Gamepads.list()
Or create a listener that allows you to listen to the keys pressed on all connected gamepads:
Gamepads.events.listen((GamepadEvent event) { });
As you can see, here we have the GamepadEvent event that has the structure mentioned before; as you can see, the use of the package is extremely simple, since it does not require establishing a connected control or something similar, we automatically have a listener of all the controls connected to the device; from the player component, we place the listener for the gamepad:
import 'package:gamepads/gamepads.dart';
***
@override
Future<void>? onLoad() async {
// final gamepads = await Gamepads.list();
// print('Gamepads' + gamepads.toString());
Gamepads.events.listen((GamepadEvent event) {
print("gamepadId" + event.gamepadId);
print("timestamp" + event.timestamp.toString());
print("type " + event.type.toString());
print("key " + event.key.toString());
print("value " + event.value.toString());
});
}
As a recommendation, connect a device to your PC/Mac, by Bluetooth or its USB cable, start the application and start pressing some buttons on the gamepad, including its joystick and d-pad and analyze the response, you will see that some are actually "button" and others are "analog" based on the type classification mentioned above, in the case of buttons, you will see that the above listener is executed when the button is pressed and released.
Based on tests performed from a Windows 11 device with an Xbox S|X controller, we have the following implementation; to detect the "A" button:
import 'package:gamepads/gamepads.dart';
***
@override
Future<void>? onLoad() async {
Gamepads.events.listen((GamepadEvent event) {
if (event.key == 'button-0' && event.value == 1.0) {
print('rotate');
}
});
}
And for the d-pad, detect the moving:
import 'package:gamepads/gamepads.dart';
***
@override
Future<void>? onLoad() async {
Gamepads.events.listen((GamepadEvent event) {
if (event.key == 'button-0' && event.value == 1.0) {
print('jump');
} else if (event.key == 'pov' && event.value == 0.0) {
// up
print('up');
} else if (event.key == 'pov' && event.value == 4500.0) {
// up - right
print('up - right');
} else if (event.key == 'pov' && event.value == 9000.0) {
// right
print('right');
} else if (event.key == 'pov' && event.value == 13500.0) {
// buttom right
print('buttom right');
} else if (event.key == 'pov' && event.value == 18000.0) {
// buttom
print('buttom');
} else if (event.key == 'pov' && event.value == 22500.0) {
// buttom left
print('buttom left');
} else if (event.key == 'pov' && event.value == 27000.0) {
// left
print('left');
} else if (event.key == 'pov' && event.value == 31500.0) {
// top left
print('top left');
}
});
}
The case of the d-pad is interesting since, depending on the plugin used, it corresponds to the same button, therefore, when pressing "up arrow" we have a value of 0.0, when pressing "down arrow" we have a value of 18000, like you can see, they are values that correspond to a space of 360 degrees; therefore, we can customize the experience however we want; finally, the implementation using the d-pad looks like:
import 'package:gamepads/gamepads.dart';
***
@override
FutureOr<void> onLoad() async {
// var gamepads = await Gamepads.list();
// print('*******');
// print(gamepads.toString());
Gamepads.events.listen((GamepadEvent event) {
if (event.key == 'button-0' && event.value == 1.0) {
// print('Rotate');
_rotate();
} else if (event.key == 'pov' && event.value == 0) {
// up
// print('up');
movementType = MovementType.up;
} else if (event.key == 'pov' && event.value == 4500) {
// up right
// print('up right');
} else if (event.key == 'pov' && event.value == 9000) {
// right
// print('right');
movementType = MovementType.right;
} else if (event.key == 'pov' && event.value == 13500) {
// buttom right
// print('buttom right');
} else if (event.key == 'pov' && event.value == 18000) {
//bottom
// print('bottom');
movementType = MovementType.down;
} else if (event.key == 'pov' && event.value == 22500) {
// buttom left
// print('buttom left');
} else if (event.key == 'pov' && event.value == 27000) {
// left
// print('left');
movementType = MovementType.left;
} else if (event.key == 'pov' && event.value == 31500) {
// top left
// print('top left');
} else {
movementType = MovementType.idle;
//
}
});
***
}
Of course, it is possible that these values will change if you use another type of control or in future versions of the plugin, therefore, the reader is recommended to adapt the above script to your needs.
With this implementation, we have other inputs for the games that we have implemented in this book and that you can of course adapt to others like the dinosaur game.
Video Transcript
In this section we are going to resolve some small final details about our game also final details that we can expand for other implementations such as the shared preference that you surely know or the Router, I hope you know it from flutter is a mechanism that we have par excellence to save user data in this case for all users it will be game data that would be for example the level and the type of game For example if I come here and select the single-level that exists I think 2 here I put level 2 I come here my visual Studio code and restart you will see that level 2 still prevails and the same with the type of game I come here I select the type of game Well I have this whole novel of name and restart it is still maintained remember here here when we select the type of game it automatically goes to level 1 something that you can change But at least this is the implementation that we carried out and this is how I left it this feature as I tell you you can implement it in the rest of the video games that we create But well apart from that we also created a couple of mechanisms to be able to interact with the game the problem that we currently had is that we need to interact with the keyboard which is not completely correct since if you want to run the mobile game then you would have to force the user, that is, the end user, you have to connect a keyboard to their Android or iOS device, a tablet, whatever they use, which logically is not correct, what we did to solve this? Well, basically here we created a virtual joystick as you can see, this is no longer provided by flame, therefore the integration was relatively simple, and here we also had a button to rotate the Player, of course, so we implemented more buttons in case it was necessary, even more joystick in case it was necessary, but well, in this case, we implemented this one, which would be the only one necessary for this game. So what else did we do? As you can see in another video that is running, attached to this one, we also implemented a gamepad to be able to move our Player, of course, at least for the moment at the time when I recorded this video. The package we used is not directly what flame tells us, which you can consult in the official documentation in the other entries section, is that this package has been out of date for a long time, therefore we used another solution, one directly for flutter, which we adapted for our application in Play. But that package has some shortcomings And it is that, it is only used on mobile, that is, Android and iOS so well This is what we are going to do in this section And remember that you can implement this same solution in the rest of the video games that we have already created and that we are going to continue creating in this course Ok before starting to work with the gamepad I wanted to make a small introduction of what we have as we are at the moment in which I recorded this video What is the ecosystem And what do we have at our disposal So for that I am going to do this small presentation I think that when you hear the word gamepad There is no doubt about it about What this component is in the same way there is Remember that you can search here on the internet and well here you will see a gamepad a gamepad is basically what we colloquially call a video game controller, that is, the next element that you can see on the screen the most famous are the Dual well the sun not the sensor or I don't know what the sound one is called this one that we have right now Here from the PlayStation 5 And of course the one from the Xbox and also the one from Nintendo switch the one from the Xbox is this one that I am going to you to show because it is the one that I am going to use in this presentation Xbox s control Well that one or x either of the two are these that we see here precisely This is the one that I am going to use But again you can use any other in case you do not have it here is where the interesting thing comes the matter for the test that at least I am going to do particularly I am only going to use one control since I only have this type of controls currently I do not have a PlayStation and I do not have any other style Although in principle with the PC recognizing it is a sufficient condition for you to be able to use it So that is basically what we are going to want to adapt to our application I think the reason is obvious The more elements we have to interact with the game we have already presented for this series third we have presented the keyboard which was always the one we used mostly we also presented the virtual joystick and this also includes what were other elements for example the button that we use to rotate our Player and now we are going to present another one that is a gamepad to see how it works and how we can use it here in play for the rest eye more to say So let's see what we currently have to be able to send it.
Flame Gamepad Plugin
So for this one I'm going to go here to the official documentation and well And here I already have the link, in the same way right now you see how you can Enter this link so that you also see what currently exists since they may change it Here I am on the latest version as is you can see the one I made and here in input the one that says other impotences and other entries here we have the joystick that you can also take a look at and at the end of everything we have the gamepad What's good is that they define it basically a line and a half in which it tells us that this works with a separate plugin and you can have more information by clicking Here we are going to see what we currently have to date, at least at the time I recorded this video. I can consider this plugin to be practically abandoned since a package for me that is more than two years old at most and depending on the package but that is how my limit is without updating and I consider it as obsolete or abandoned, this one for example is about three years old, at least three years old. Here you have the date of the last commit that is not updated and it is the one that the people from frame are recommending here. then Well it doesn't convince me, try to install it and I had problems with the version dependencies and everything else. Therefore, I had to lower the versions that I currently had in order to use it and logically I don't want to do that. Then Well, if you want to use this package, you will surely have some problems and well, I don't know how it will work in the future, they will continue to update. But at least at the moment, in the degree of this video, I consider it to be practically abandoned. Remember that at least three years ago, and as of today, it is 2023. Three years ago, Blue safety didn't even exist, so well, you may have problems there. But currently, to analyze a little, it says that it works with Android, for the moment, for the rest, its use is as expected. Simply here we detect the connected device and start listening to the buttons and well, the plugin that we are also going to use works in a similar way. So with this, we can leave this page and continue here, advancing a little in my book, a fragment of my book, then you can do a search. In this case, I would not recommend that you put flame because only those two pages will appear, but directly in, since we saw that we can also use several packages or simply flutter syntax as such. that is to say relative previously we saw how to use the one that we have here now it is here to see preference that well is here in my menu but I don't see it that it is an exclusive package for flutter but we could also use it without any problem you could say for Play and here we have another one that is the one that I found is that it seems to me that it looks better to say that it looks better although it is also perfect it is in development there is barely the version that we currently have at the time in which this video is recorded you surely already had a higher one when you see this video and it is in development therefore since it is something quite recent it is in development the official documentation is quite scarce or to say non-existent at least currently and another detail is that well the documentation that we have here is also a bit abstract that is to say what is completely clear how it works But well for that is what we are going to make the video in which we are going to use This plugin So what do we have here to explain to you a little how the plugin works if we come here Well let's see the reference part the one we have here basically we have is although I still can't find the page that interests me I'm going to look for it a little I think it was here
How the gamepads plugin works
Ok perfect we have these three elements one is the gamepad controller notice that we are talking about classes the gamepad controller refers to the control as such that is to say the gamepad the gamepad already refers to an input that is to say this class It is automatically built by Well this package as we are going to see for a few moments and what it returns to us is an event an event of type gamepad which again corresponds to the input Here we have information as you can suppose to see if it enters through here although it was not this one that I wanted to see it is here it is here it is better the ID since we can have multiple controls connected to the device in Android whatever and Well but here we are going to listen or we are going to identify it by gamepad ID is zero one two three whatever if you have two controls you are going to have zero and one surely since they are that it seems a little crazy to me but it is the date on which the key was triggered to press that is to say if it was at the moment in which the type that this could be or button or directly was pressed Well let's see it since Element is also around here Although Well it is going to present it to you here better so as not to navigate too much it could be button or the analog, the analog refers for example to the styles, that is to say the levers and also the crosshead, the buttons would be the rest, for example in the case of the Xbox it would be the bird and x button And this is what it gives us back here in the type We also have here which is another very important element that refers to the key in this case it is also like a type since for example at least in the case that I tried with this control, the one from the Xbox that I mentioned to you, the one from here refers to it.
For example if I press the key it returns something like button-0 and well something like that for the rest and it is also a very interesting element since it depends on what you are pressing For example if you press a button Then when it is pressed it sets the value of one and when you release it it sets the value of Zero and something similar to the rest of the elements But well I think we see this better in practice so for now I will leave it there But this is what we have So how do you eat all this coming back here here we also have the gamepad which well they don't put a description but in the end it is also a kind of utility that basically allows us to put together these two and well seeing some code and for that we can present it My goodness I found something here in the examples section well This is not really understood this is an example Well it is not that it is not understood But it is quite abstract it is not what I want to show you Although Well you can run it there and you see how it works but it is not the example that I want to find that is why I tell you that here the documentation is nonexistent and a little difficult to keep going back Here is this page to which it is the documentation I think was the one that appears here when we give preference if it is right here we have the gamepad Which notice that we can interact or here All this begins it returns a list of the devices For example if we have a control connected Then it will not return a root with control if we have two it would return 2 etcetera of course controls in this specific case that recognizes the device specifically in my case my Windows machine that we have well that the ones I am recording I have to have a control connected to me in particular it worked for me by connecting the Bluetooth but if it does not work for you by Bluetooth you can also connect it by cable This is the interesting one and it is the one that we are going to use Note that simply two lines of code that is the one that triggers the events, that is to say every time we press a button or a crosshead an analog one over here this method will be executed and over here we will have information and here we have the event the event that refers to there that will show you over here Well I am going to come back here gamepad even with all the information that I mentioned to you before is what we have here here I tell you that it is a little abstract because well they do not signal it well A my opinion they don't even make reference to that class here they simply indicate even and that's it. So even when you make the breakpoints there they don't work well, we'll see that in detail but anyway here is what we have for here also if it tells you that the event but anyway It seems to me that this is a bit disorganized for the rest Note that it is not necessary to register it, it is not necessary to register that one, it is not necessary to register the device or anything like that it is extremely simple, extremely clean what we have to use. So this is the plugin that we are going to use, specifically we are interested in this function:
Gamepads.events.listen((GamepadEvent event) {
});
But well let's practice a little more and we'll also see what this function returns to us then finally To install it to get out of this is the typical thing we put gamepad Well whether I execute that Command whatever I'm going to return here I put an important point is that this plugin does not work for mobile phones or web therefore you can not use it there in principle I understand that it works for PC in this case Windows I have not tested it in math but that it also works more and of course Linux But well there that is the one we have at our disposal at least for the moment since there are really very few packages that work with gamepad specific for of course for frame only exists or at least that I know the one that I presented to you initially so for the rest little more to say again it works for Windows and I think for more Linux I have not tested it the package is very simple simply here we have a method to obtain the connected controls others to listen to the pulsations to the analog or the buttons This is the event that describes us and little more to say install it and with this we can continue to the next video.
Of course if you are interested in doing this implementation you have to have a video game controller that recognizes your PC with you because if you don't have it you will not be able to test the implementation Ok here we have our video game you can also see that I activate the camera in this case it is focusing on the control that I am going to use to do this test as I was telling you it is an Xbox s controller any other should work for you but well if you are a gamer or have played some video games on PC you should know that even for more robust games, that is to say using more robust technologies there are even problems with the controls so well don't expect that it will be different here then Well here this is important if you live with a relative or something like that a girlfriend or something remember to tell her that you are working with me that you have a PlayStation controller or whatever in your hands well having said that Now yes we are going to begin as I indicated the extremely simple implementation Here also remember to have placed the plugin that has been installed
dependencies:
flutter:
sdk: flutter
***
gamepads:
So we are going to work specifically here on the Player component in the event called onload that we have somewhere else but in the init Well here we have it perfect I'm going to place it up here really because it matters where you want to place it But well here that we have a like this we can take advantage of it So here what we are going to do is see what devices we have connected for that Here I am going to create a variable called kimbax which is the same as a wave since well as you can imagine it is an asynchronous operation to gamepad here we have a package would be this one for gamepad gamepads point ready and it is that simple now with this we have access to all the controls that are connected this again is a test we are not going to implement anything yet here I am going to print it to see what it prints to see if it is similar to what the official documentation showed and here already To take advantage of the time we are going to define our gamepad This is for the listener event you see and here we place the would be the here I like to put the typing so that it is clear I am going to call 9 in the official documentation it only appears like this But again I like to place it with the type this by the way is a function that is to say the argument is a function So we put it inside some parentheses and here in what we have then here we can place a print or something like that and Well a little more to say here I would also like to always like for the prints to place something striking to be able to locate myself better I put this here and this also here Although in principle Remember that this event since it is an event has all this available what I was telling you there the device the date which exists the press the type if it is analog or button the button as such what it places There is a typing I don't know why not use it in an enum But well they left it like that and here in value if we save I am going to learn the control I am going to leave it here pressed there it turned on I am going to wait a few moments Let's see what happens and I am going to update the application here look at what we have in this case I have it connected by bluetooth to my PC and here it takes it perfectly here the instance of the connected control appears if I turn it off how good to pay this is a dilemma I am going to see if it wants to turn off if not I remove the battery there turned off and reload the application sometimes it appears because well it takes a while here it doesn't have it to detect that it's not present then you can see that this is the controller that is working here you can see that this event also works a little strange since when it recognizes it then there are times that it runs too and it gives you this bunch of prints this Remember that it refers to a button pressed although I didn't put any the section of the middle one that I don't think it registers but well sometimes this happens I don't understand very well why but that doesn't really matter then here again we have the gamepad controller control which was one of the three main classes that I told you that we had well that we have available in this package so really with this we're not going to do anything but for example here you can ask for elements like the ID then Well remember that this is a list So I ask for position 0 here we have the gamepad controller and here we have the ID here it tells me that it is an Xbox controller as you can see and well some additional information but the important thing here would be Lady and the name in case you want to place it somewhere in the game there you have it then I'm going to here to reload everything here in this point you have to have the window active, that is, focus, because if you press here the control will not recognize anything here
0 and 1 by pressing the buttons
I am pressing as you can see and nothing happens Now at this point you can comment So that it does not make noise and you can see that it does not work if you do not have the window active So you place the window here and here you will see what appears notice when I leave it pressed, put the value of one Well here it closed it because I lost focus when I press a button put the value of one here it will release it and put the value of Zero and the same for the rest here I pressed and notice that it is button zero well my God what a maniac to do this the button zero for this one which is the B button 1 the same one active zero deactivated This is button 2 and well You have to map this yourself to say I don't know if this changes depending on the control you are using or if they are going to change it in the future etc. you have to keep in mind these values the joysticks are a little bit well not to say that it is poorly implemented because I don't think it is but quite complex look that as soon as I move it look that here we have values if I move it enough here you can see how the values change and here it also changes the button name and the same for the other one well This is interesting because logically the less the movement then the smaller the value The longer the movement as in this case I am stuck to the end The value should be greater as you can see for the rest we have the same for the rest of the buttons this would be for the stay and the Select we have values just like over here what we have behind there we have them and
Crosshead, variable values
The crosshead is also interesting, look if I put it up here we have the value of zero, if I put it here is that I have it the other way around in this case this would be the right it would be 9000 if I put it and right at the same time look we have 4500 if I put it down we have 18000, that is, I think you noticed but here we have a value that is similar to a 360 degree radius again or it would be zero, Button would be 18000 by it would be 9000 and for left it would be 27 and again left would be well to see 31,500 so we have to keep those values in mind for when we want to Listen here to these buttons as is you can see what the idea is. Well basically being able to move the Player here in this case it will move it with the keyboard or however you want, we can see that it only moves in a straight line, that is, up, down, right and left, if you use the keyboard it does not move laterally like that, in this case it does move laterally because we are using a…
Joystick
Virtual joystick but for the implementation that we did for the keyboard they don't do it. Of course here you could implement this as you want but well here what I'm indicating is what we have here for example for the crosshead which is what we are going to use to move this Player we can even detect inclined movement here To put it in some way that is to say pressing op and left or up and right at the same time or right button at the same time therefore there I could take advantage of it to move it in an inclined way I'm not going to do it like that the implementation because It's simply not what we did here Remember that the movement for the keyboard event would be what we have here right therefore here you would have to implement another logic of which you place the inclined ones there that is to say and well but well I don't want to do it because I simply want to take advantage of the code that we currently have for this small project for this small adaptation so well this would be practically everything In short, once the plugin is installed, place the event listener here right here in the Player components as we have it here we have the Well we are printing the event So here do some tests identify which are the buttons that you want to use for achieve the movement in my particular case is going to be the button a to do the rotation and the crosshead to do the movement the rest does not interest me but well feel free to finish adapting it For example if you press here they are that you put pause here or display some menu those small adaptations feel free to do them as you consider best so little more to say before finishing if I would like to place a breakpoint here although it does not pay attention to me I go here to reload there it is and well if you evaluate it here you will see what it returns to you This then we do not have information there but anyway remember here also important that you can put for example gamepad ID and this I take it automatically thanks to the typing that we put here if you do not put it then Well here it recognized it I do not know why but well most likely none of this will appear to you if you simply put although well everything depends on the version that you are using but well it was so that you also had it in mind here I know ID we save I am going to remove this breakpoint I am going to reload again here the active screen and you press and there you have it again in summary try here get the state just as an exercise just listener here you can put one property or another so put whatever you want active window and start pressing the keys here well the buttons so you can see what appears here also feel free to put the rest of the values that would be something like this so you can get more information here I already had it here a cheat sheet If we restart here I press the crosshead here you will see that it says it is of analog type if you press a button it is of button type and well here again the values that the values you can use depending on the type b button or analog that you are using as you can see again for the buttons it is 0 and 1 for the crosshead it is something a little more interesting that we can also take advantage of to know what the inclination was Or what exactly was put here by the way it also works in a similar way when you press it it keeps the value and when you release it it puts another value that I really don't know what it refers to But well what appears there as I tell you none of this is indicated by the official documentation they are things that we have to do they are tests that we have to do.
So again to summarize install the package put listener then put these values so you know exactly how it works press some buttons the note in case you have a different definition than mine What are the buttons the name of the buttons and well for the rest there is little more to say once this is done we can make the implementation very but very simple but that will be the subject of the next video OK now we are going to carry out the implementation that we see here where we have a listing to listen about our game:
Gamepads.events.listen((GamepadEvent event) {
});
The keys or buttons of our controller and remember that we now have to map it, that is to say the zero button for example when it has the value of one that is when I press it we want it to rotate and the same with the rest of the buttons and analog or well I do I simply say buttons for the entire controller but well define it as you want remember that here in the plugin it says analog for those that return a value other than zero or zero and one and the buttons would be 0 and 1 again 1 when pressed and zero when released Ok one thing is also interesting is that for some reason the plugin disabled the buttons of the well the events to listen to the keyboard keys So I can't move it with the keyboard in the official documentation I found nothing then I guess it's a small Boot in the same way Remember that we are carrying out experiments and little else then Well there are several ways to do it for example if we do not have controls Then do not run this or enable it from the menu There are many ways to do it here as I tell you we are only carrying out the experiments but if it is a question of the package it seems to me Because when it runs a web if it works for me again the keyboard was good it was for say it in case the same thing is happening to you as I hope they solve it in the future anyway having said that Now yes we are going to start having all this clear I am going to comment on it and the only thing we have to do here is to make the conditions remember that in case they vary since I only have control and of this type therefore I am going to place are the values that appear to me but if others appear to you you have to place yours anyway placed here to obtain the name that I have placed to That button over here then we want in the button zero but not only this but the Evening is equal to one point zero or simply one if this is the case then here we apply the rotate for now I leave it like this to help me a little with the messages and now we place the real implementation So the next case would be I am going to duplicate this several times since it is more or less the same the next case the event would be for the crosshead or the depata they also call it So here it is not Pluto So I am going to select it but it would be the deploy to see how many I have one two three well it is going to select them all I put and here we do vary the value according to what we want to select So for the first one that would be Ok as we indicated we put 0 and here I put, remember that it is 360 degrees But well something similar a similarity that they did here the next would be the raid it will simply place it by leaving it here but we are not really going to use it as we mentioned before that it would be 4500 which would be the all right and here I also place it the next would be directly write by write the next would be 13,500 which would be button write and this would be 18000 And well we are almost there the next would be 22,500 which would be soccer the next would be Left 27,000 and the next and last Oh no We are good now the next would be top left which would be 31,500 no more is needed this would be Here also the comment and there we are I am going to reload and test well I bring the game here there we have it rotate I am going to start up tilted by right button left Ok there is one that is not working for me taking it to the left the led is not taking it let's see here it is Oh excuse me I have a little dizziness I'm going to recharge there it is perfect then it works correctly what we do now is very simple we make the corresponding call here here we rotate and here what we have to do is use the momentive is equal to ventate point this would be the let's see there it is:
Gamepads.events.listen((GamepadEvent event) {
if (event.key == 'button-0' && event.value == 1.0) {
print('jump');
} else if (event.key == 'pov' && event.value == 0.0) {
// up
print('up');
} else if (event.key == 'pov' && event.value == 4500.0) {
// up - right
print('up - right');
} else if (event.key == 'pov' && event.value == 9000.0) {
// right
print('right');
} else if (event.key == 'pov' && event.value == 13500.0) {
// buttom right
print('buttom right');
} else if (event.key == 'pov' && event.value == 18000.0) {
// buttom
print('buttom');
} else if (event.key == 'pov' && event.value == 22500.0) {
// buttom left
print('buttom left');
} else if (event.key == 'pov' && event.value == 27000.0) {
// left
print('left');
} else if (event.key == 'pov' && event.value == 31500.0) {
// top left
print('top left');
}
});
Ok let's go with the next ones again I'm not going to use this one I'll leave it there as a reference feel free to adapt it this would be for right this would be for the button or the down I don't know how to place this would be for the left I'm going to comment on all this and that's it Ok of course Here is the problem in that we always remain in motion things that would also be interesting to implement a game like this or at least a level for a little slower I could do it but there well Of course because we are always leaving the momentive here therefore we are always indicating that it is moving and there the problem here finally we could place this one is up to the doctor well we could place one two in which it would be if we press anything else let's see how it goes for us like this here we place equal to momentive point there really when we are pressing any button we are indicating that this one should be executed But well it is already in the United States you could also ask here because that is what interests us is really this except for the first one Of course but we could place it will leave it for reference so that it is clear in case you want to implement another button in case you have any conflict but what we are interested in is to ask here for zero they should evaluate it is zero and it should be of type this I think it should be Exactly the same as no no of course it will not work because this is the top here I got tangled up that it was with the button but it is not right so anything remember to leave this conditional last if you want to place more conditions you place them on top and this would be the last one obviously because it is the case it does like the one in the switch and there we have the mobility using the gamepad and with this we finish the implementation of the gamepad for our game.
I agree to receive announcements of interest about this Blog.
We will see how to use Xbox S|X or Playstation controls in a Flutter project.
- Andrés Cruz