Flame's module called GameLoop or game loop is nothing more than a simple abstraction of the game loop concept. Basically, most of the games are based on two methods:
- The render method takes the canvas to draw the current state of the game.
- The update method, which receives the time (delta) since the last update and allows to go to the next state, i.e. update the game state.
The Game Loop can be considered the most important process in video game development; since, from it is that we can implement our application.
The GameLoop, as you can guess from its name, is an infinite loop that is responsible for updating the state of the game; this goes from adding elements on the screen, moving the player or the enemies and in short, any change on the screen is done from the GameLoop; in Flame, it is basically the update() function that is executed infinitely, and it is here, where checks of various types such as listeners, etc. are placed. This loop is responsible for the game running properly; using primitives we can control the execution speed of the game so that it runs correctly, and in the case of Flame, so that it runs at the same speed on all devices; in other words, how many updates per second will occur; this is important since the number of times per second that this function is executed depends on the processing speed, and having the application running in different environments, it is important to carry out this type of configuration.
In general, the Game Loop is responsible for processing everything that happens in the game and updating the graphical user interface accordingly.
In Flame we have a method to initialize the game and another to perform updates; Flame follows these principles and we have a couple of functions that allow us to perform such operations.
Render function
The render() function receives an object type parameter that refers to the canvas:
@override
void render(Canvas canvas) {
canvas.drawRect(squarePos, squarePaint);
}
Which is just like with other technologies like HTML5, it's nothing more than a blank canvas to draw on; here, we can draw anything, for example, a circle:
@override
void render(Canvas canvas) {
canvas.drawCircle(const Offset(10, 10), 10, BasicPalette.red.paint());
}
Update function
The elements that are in the game need to be constantly redrawn according to the current state of the game; to understand this more clearly, let's see an example:
Suppose that a game element represented by a sprite (an image), which in this example we will call as "player"; when the user clicks on a button, then the player must update its position; this update is applied in-game by a function called update().
As we saw in the example of the moving circle, it is this function that is responsible for updating the position of the circle on the screen.
The update() function is as its name indicates, an update function, which receives a parameter called "deltatime" (dt) that tells us the time that has elapsed since the previous frame was drawn. You should use this variable to make your component move at the same speed on all devices.
Devices run at different speeds, depending on their processing power (that is, depending on what processor the device has, specifically, the frequency at which the processor works), so if we ignore the delta value and just run everything at the maximum speed that the processor can run, ie the game might have speed issues to control your character correctly as it would be too fast or too slow. By using the deltaTime parameter in our motion calculation, we can guarantee that our sprites will move at whatever speed we want on devices with different processor speeds.
When updating any aspect of the game through the update() function, it is automatically reflected through the render() function and with this, the game update at a graphical level.
The Game loop is used by all implementations of Game classes and their components:
https://docs.flame-engine.org/1.6.0/flame/game.html
This article is part of my complete course and book on game development with Flame.
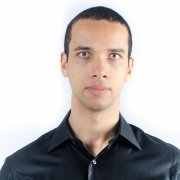
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter