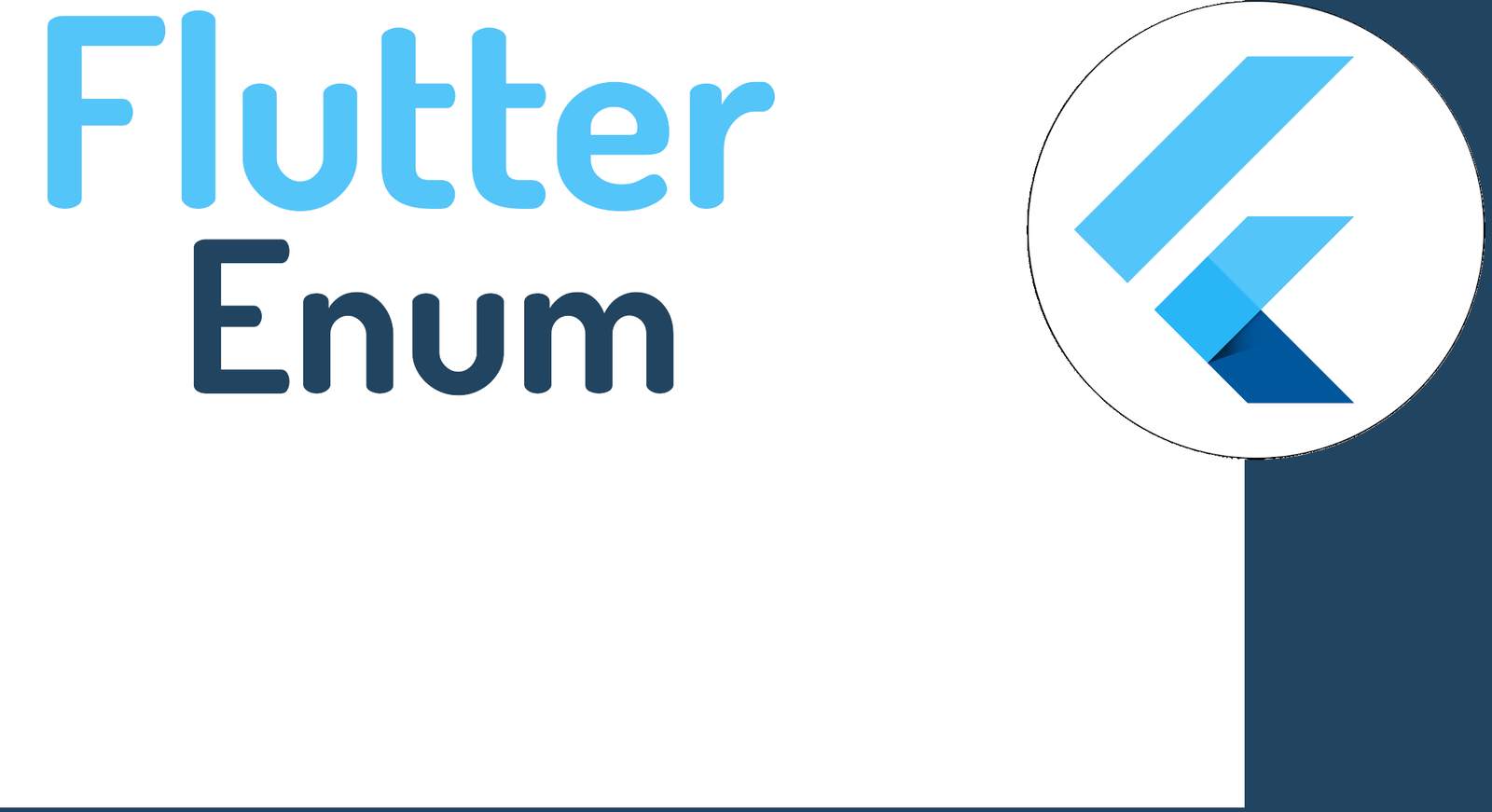
An enumerated data type (also known as "enumeration" or "enum") is a data type that consists of a set of possible values, which are clearly enumerated in the type declaration. Instead of representing a value (such as an integer), it is represented by an array of key/value values. Enumerated types are a useful way of doing constant sorts in your application.
In this article, we discuss how we can extend the functionality of our Enum using extension methods.
Let's create Enum
enum BottomTabEnum {
home,
video,
shop,
profile,
}
The Enum we created above is for the bottom tabs of our application. We use this Enum for our bottom navigation bar. We have 4 tabs in our app. We will now add more purpose to this Enum using extension methods.
extension BottomTabsExt on BottomTabsEnum {
String get title {
switch (this) {
case BottomTabsEnum.home:
return 'Home';
case BottomTabsEnum.video:
return 'Explore';
case BottomTabsEnum.shop:
return 'Shop';
case BottomTabsEnum.profile:
return 'Profile';
}
}
String get iconAssetLocation {
switch (this) {
case BottomTabsEnum.home:
return Assets.imagesChefCooking;
case BottomTabsEnum.video:
return Assets.imagesVideoCamera;
case BottomTabsEnum.shop:
return Assets.imagesOnlinePayment;
case BottomTabsEnum.profile:
return Assets.imagesWomanUsingPhone;
}
}
Color get bgColor {
switch (this) {
case BottomTabsEnum.home:
return const Color(0xFFEDC35D);
case BottomTabsEnum.video:
return const Color(0xFFF59273);
case BottomTabsEnum.shop:
return const Color(0xFFE1EFEE);
case BottomTabsEnum.profile:
return const Color(0xFFF2BBBB);
}
}
onClicked() {
switch (this) {
case BottomTabsEnum.home:
///Handle onClick
break;
case BottomTabsEnum.video:
///Handle onClick
break;
case BottomTabsEnum.shop:
///Handle onClick
break;
case BottomTabsEnum.profile:
///Handle onClick
break;
}
}
}
We have created different extension methods for our Enum. This will simplify our code greatly.
Let's use our Enums in an app in Flutter
///To get the title
Text(BottomTabsEnum.video.title);
///To get the icon asset location
Image.asset(
BottomTabsEnum.home.iconAssetLocation,
width: 18,
height: 18,
);
///To get the bg color
Text(
'Cool extension',
style: TextStyle(
color: BottomTabsEnum.profile.bgColor,
fontSize: 14,
height: 1,
fontWeight: FontWeight.w600,
),
);
///To perform click action
GestureDetector(
onTap: () {
BottomTabsEnum.home.onClicked();
},
child: child,
);
With the example above, you can see that there are endless possibilities. We can assign more functions to our Enum constants without creating additional classes.
https://arkapp.medium.com/supercharge-enum-with-extensions-in-flutter-abf8fdf706fe
I agree to receive announcements of interest about this Blog.
We will know how we can use the enum extension in Flutter.
- Andrés Cruz