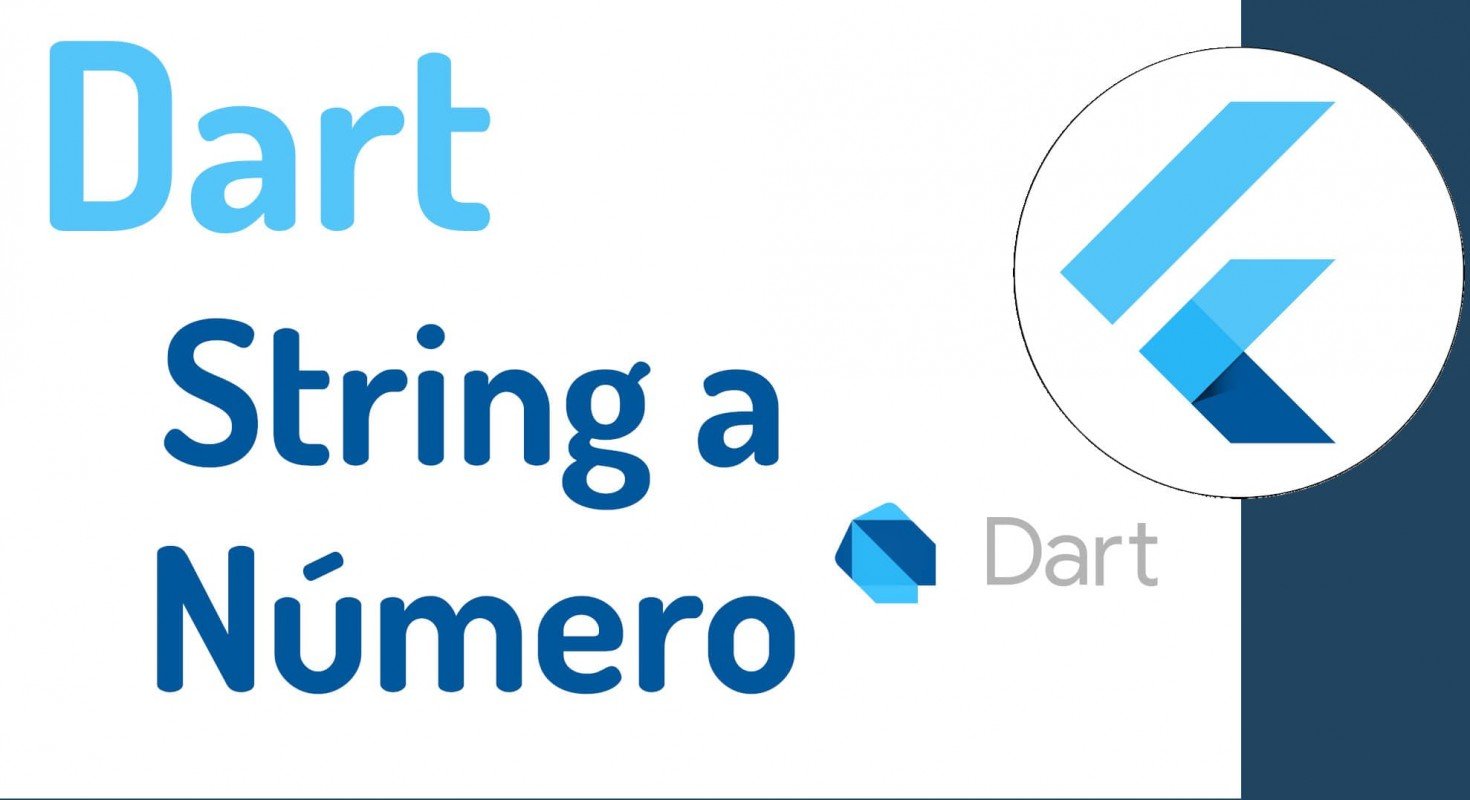
In this post, we learn some methods to parse/convert String to a number (integer, double, or both).
1. Use the parse() method (not recommended)
int stringToInt_parse(String input) {
return int.parse(input, onError: (error) {
return NULL;
});
}
double stringToDouble_parse(String input) {
return double.parse(input, (error) {
return NULL;
});
}
num stringToNumber_parse(String input) {
return num.parse(input, (error) {
return NULL;
});
}
Output
print(stringToInt_parse(testInt)); // 100
print(stringToDouble_parse(testInt)); // 100.0
print(stringToNumber_parse(testInt)); // 100
print(stringToInt_parse(testDouble)); // NULL
print(stringToDouble_parse(testDouble)); // 100.1
print(stringToNumber_parse(testDouble)); // 100.1
print(stringToInt_parse(testInvalidNumber)); // NULL
print(stringToDouble_parse(testInvalidNumber)); // NULL
print(stringToNumber_parse(testInvalidNumber)); // NULL
Notes:
- We can use double.parse() to convert a valid integer string to an int, but it will return a double (Line 7: 100.0). And obviously we can't convert a valid double string to an integer, it will return null (Line 10: null).
- With num.parse(), the program will try to parse the integer first, if it can't parse (get null), then it will try to parse double. (That's why on line 8, the output is 100 but not 100,0.)
- I don't know exactly the reason behind this, but if you look at the signature of int.parse(), double.parse() and num.parse(), you'll see that onError is an optional named parameter to int. parse() while with double.parse() and num.parse() it is an optional positional parameter.
2. Use the method tryParse()
When we use the tryParse() method, we only need to provide the input string. In case the input is not valid, the program will return a null value. This is the difference between tryParse() and parse() (which throws an exception if we don't handle the onError method).
int stringToInt_tryParse(String input) {
return int.tryParse(input);
}
double stringToDouble_tryParse(String input) {
return double.tryParse(input);
}
num stringToNumber_tryParse(String input) {
return num.tryParse(input);
}
Try
final testInt = '100';
final testDouble = '100.1';
final testInvalidNumber = 'a';
print(stringToInt_tryParse(testInt)); // 100
print(stringToDouble_tryParse(testInt)); // 100.0
print(stringToNumber_tryParse(testInt)); // 100
print(stringToDouble_tryParse(testInt)); // 100.0
print(stringToDouble_tryParse(testDouble)); // 100.1
print(stringToNumber_tryParse(testDouble)); // 100.1
print(stringToInt_tryParse(testInvalidNumber)); // NULL
print(stringToDouble_tryParse(testInvalidNumber)); // NULL
print(stringToNumber_tryParse(testInvalidNumber)); // NULL
3. (Bonus) Use extension methods
In case you don't know what extension methods are, it's a way to add more functionality to existing libraries/classes. In our case, we use this technique to add 3 methods to the String class.
extension StringExtension on String {
int toInt() {
return int.tryParse(this);
}
double toDouble() {
return double.tryParse(this);
}
num toNumber() {
return num.tryParse(this);
}
}
After creating the above extension, now our String has 3 more methods that we can use as other output methods.
final testInt = '100';
final testDouble = '100.1';
final testInvalidNumber = 'a';
print(testInt.toInt()); // 100
print(testInt.toDouble()); // 100.0
print(testInt.toNumber()); // 100
print(testDouble.toInt()); // NULL
print(testDouble.toDouble()); // 100.1
print(testDouble.toNumber()); // 100.1
print(testInvalidNumber.toInt()); // NULL
print(testInvalidNumber.toDouble()); // NULL
print(testInvalidNumber.toNumber()); // NULL
I agree to receive announcements of interest about this Blog.
We are going to know how we can pass String to integer or real numbers using Flutter, specifically Dart.
- Andrés Cruz