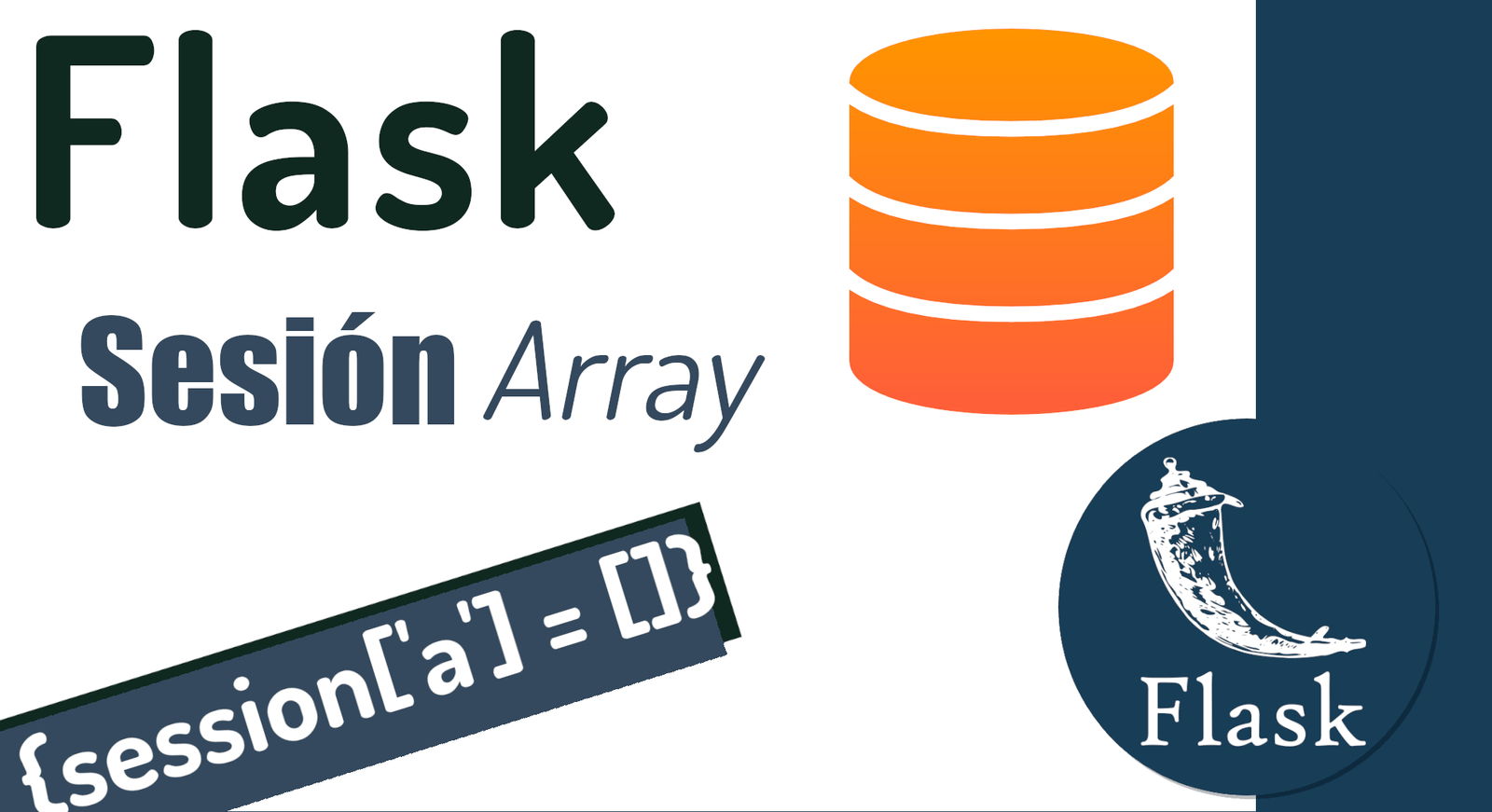
In Flask, the session is a way of storing data that is associated with a user during the use of the application, usually the use of the session is related to an authenticated user, but the user can also use the session without having to be authenticated. For example, if a user logs into a Flask web application, a session can be created to manage the basic data of this user, such as her name, email and indentifier, among other preferences.
Flask provides an easy way to work with the session through the session object, which is a Python object that is stored in cookies in the user's browser.
To use the session in Flask you can use functions like session.get() and session.pop() to read and write data to the session, respectively.
The use of the session is very simple in Flask and we will see how we can save more than just text, numbers or the like:
session['age'] = 30
session['name'] = "Andres"
First of all, remember that we can import it internally from Flask:
from flask import session
And we define a couple of functions, to add and remove elements from an array, in this case:
def addRoomSession(room):
if 'rooms' not in session:
session['rooms'] = []
rooms = session['rooms']
rooms.append(room)
session['rooms'] = rooms
print(session['rooms'])
def removeRoomSession(room):
if 'rooms' not in session:
return
rooms = session['rooms']
rooms.remove(room)
session['rooms'] = rooms
The idea is to notice that we can store arrays inside the session:
rooms = session['rooms'] # get the session, the key we want to modify
rooms.append(room) # we add our elements
session['rooms'] = rooms # set it back to the session
This code is part of my complete course on Flask, in which we see how to work with Flask Socket IO and rooms.
I agree to receive announcements of interest about this Blog.
We will see how to work with the session type array in Flask.
- Andrés Cruz