Before we saw how comunicar el proceso de rencerizado con el proceso principal
To pass the data defined in the main process, we are going to use the following structure; the data will be available from the main process, to simulate an external structure such as a database, we are going to create a separate file for index.js, which is the one that will be in charge of supplying the data, we will create a couple of modules with the data so that they are easily consumable:
data.js
const contacts = [
{
'name': 'Alex Alexis',
'image': 'https://randomuser.me/api/portraits/women/56.jpg',
'last_chat': [
{
'date': '9:15 AM',
'message': 'Lorem ipsum dolor sit amet',
}
]
},
{
'name': 'Eli Barrett',
'image': 'https://randomuser.me/api/portraits/women/96.jpg',
'last_chat': [
{
'date': '8:30 PM',
'message': 'Lorem ipsum dolor sit amet',
}
]
},
{
'name': 'Kylie Young',
'image': 'https://randomuser.me/api/portraits/women/45.jpg',
'last_chat': [
{
'date': '8:30 PM',
'message': 'Lorem ipsum dolor sit amet',
}
]
},
{
'name': 'Kylie Young',
'image': 'https://randomuser.me/api/portraits/women/45.jpg',
'last_chat': [
{
'date': '8:30 PM',
'message': 'Lorem ipsum dolor sit amet',
}
]
}
]
const chats = [
{
'user': {
'name': 'Alex Alexis',
'image': 'https://randomuser.me/api/portraits/women/56.jpg',
},
'chat':
{
'date': '9:15 AM',
'message': 'Lorem ipsum dolor sit amet consectetur adipisicing elit. Doloribus reprehenderit voluptatibus cumque, deserunt deleniti consequatur adipisci nisi consequuntur sunt itaque? Sunt aspernatur, ratione labore ipsam enim unde itaque dolorum magni?',
}
},
{
'user': {
'name': 'Eli Barrett',
'image': 'https://randomuser.me/api/portraits/women/58.jpg',
},
'chat':
{
'date': '9:50 AM',
'message': 'Lorem ipsum dolor sit amet consectetur adipisicing elit. Doloribus reprehenderit voluptatibus cumque, deserunt deleniti consequatur adipisci nisi consequuntur sunt itaque? Sunt aspernatur, ratione labore ipsam enim unde itaque dolorum magni?',
}
},
]
module.exports.contacts = contacts;
module.exports.chats = chats;
From the index, we activate the integration with Node and consume them; We define an event in which, when loading the window (web page) through the did-finish-load event, we transmit the data via a message:
index.js
const { chats, contacts } = require('./data')
***
function createWindow(){
let win = new BrowserWindow({
width: 1300,
height:900,
webPreferences:{
nodeIntegration: true,
contextIsolation: false
}
})
win.loadFile("index.html")
win.webContents.openDevTools()
win.webContents.on('did-finish-load', () => {
win.webContents.send('pr-chats',chats)
win.webContents.send('pr-contacts',contacts)
});
}
From the web page, thanks to the fact that we activated the integration with Node, we can import the ipcRenderer module, in order to communicate with the main process, specifically, we are interested in creating a listener (listener) to receive the data sent from the main process:
index.html
<script>
const { ipcRenderer } = require('electron')
function createContacts(contacts) {
var lis = ''
contacts.forEach((c) => {
lis += `<li class="p-2 card mt-2">
<div class="card-body">
<div class="d-flex">
<div>
<img class="rounded-pill me-3" width="60"
src="${c.image}">
</div>
<div>
<p class="fw-bold mb-0 text-light">${c.name}</p>
<p class="small text-muted">${c.last_chat[0]['message']}</p>
</div>
<div>
<p class="small text-muted">${c.last_chat[0]['date']}</p>
<span class="badge bg-danger rounded-pill float-end">1</span>
</div>
</div>
</div>
</li>`
})
document.querySelector('.contact').innerHTML = lis;
}
function createChats(chats) {
var lis = ''
chats.forEach((c) => {
lis += ` <div class="d-flex chat">
<div class="w-75 ">
<div class="card bg-dark">
<div class="card-body text-light">
${c.chat.message}
</div>
</div>
<p class="small text-muted float-end">${c.chat.date}</p>
</div>
<div class="w-25 d-flex align-items-end">
<img class="rounded-pill ms-3 avatar" src="${c.user.image}"/>
</div>
</div>`
})
document.querySelector('.chats').innerHTML = lis;
}
ipcRenderer.on('pr-chats',(event, chats)=>{
createChats(chats)
})
ipcRenderer.on('pr-contacts',(event, contacts)=>{
createContacts(contacts)
})
</script>
Remember that the previous material is part of mi curso completo sobre Electron.js
- Andrés Cruz
En español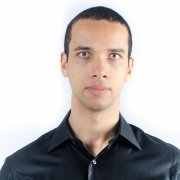
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter