In this post we are going to see how we can create what in computing is known as "Hello world" in Django, what it means to take the first steps in any programming language, framework, etc. and with this, learn the basics of this tool with a simple example. .
In this entry we are going to know how we can work with two layers of our MTV in a basic way, which has been the layer of the View and the Template, in addition to this of another layer that has been the one that allows us to route, which is a layer intermediate.
Creating our first function in our app's view in Django
To do this, remember that you have to have your Django project installed and created and your application created beforehand, which we already did in previous posts.
We are going to go to:
yourProject -> yourApplication
Which has been within your project and then within the application that we created in the previous entry:
firstProject/firstApp/views.py
Here's everything you need to boot up your Hello World web app with Python.
A Django app is simply a Python package that follows a certain file schema and base structure that we can use for our Django web projects.
We are going to open the view file called views.py that is inside our firstApp application.
As you can see, it is a completely empty file and in which we can define different structures at the class and/or function level to be able to develop some functionality (such as a list, process a form, send an email, etc.) and that it can be consumed. by our user through a route; We are going to go for the easiest thing that would be to create a function that can take any name, any name allowed by Python of course:
from django.http import HttpResponse
def index(request):
return HttpResponse("Hello World")
As you can see, we don't have anything too complicated; just a function called index, although you can name it whatever suits you best, and it is returning something, this something has to be a valid HTTP response as it remembers that we are developing a web app so all we have to answer is a HTTP response. We use the class called HttpResponse to create the response which is only going to make a complete Hello World example.
Create routing
Now, we have a sample function that is ready to be consumed by our browser, specifically by a URL or path; so now we have to explain a new layer that will allow us to create this routing.
We are going to create the route so that it can be consumed by our browser specifically through a route or URL;for that we are going to create a file called urls.py to the urls.py file INSIDE our application:
firstProject/firstApp/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
If you've worked on other modern frameworks like Laravel or CodeIgniter 4, this will be very common for you; first we are importing one of our layers represented by views.py and then either we are creating an array of routes, which at the moment we only have one route, therefore it is an array of one position; in which we are indicating the path, then the function that is in charge of resolving the path and we are giving it a name that is completely optional and in another entry we will see what it is for us to define a name for a route.
Register our application's routes file in our project
Now we need to go to the module inside our project that has the same name as our project and that file is called urls.py and we need to register the routes file that we created earlier for our application.
from firstApp import urls
urlpatterns = [
path('fisrtApp/', include('firstApp.urls')),
]
View our Hello World in the browser
In order to finally be able to do all our work, we have to execute the following command at the height of your project (where a file called manage.py is located):
python manage.py runserver
And with this, if we go to:
http://127.0.0.1:8000/fisrtApp/
We will see the Hello World with the following result:
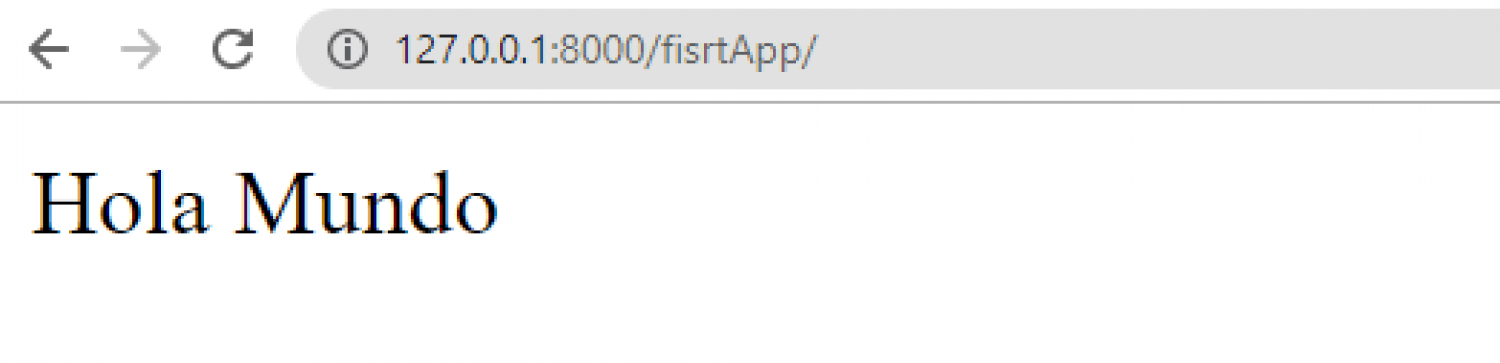
Conclusions
As you can see, our application is fully functional and ready to do something more interesting than the one shown in this example, which we will do in later posts.
Remember that if you were left wanting more, you have my course in Django 3 that you can obtain more information in this blog in the links in the header.
Hello World
In this section, we are going to create a Hello World, for our application; let's start by creating the following function, which is what we are going to consume through the browser using HTTP:
comments\views.py
from django.http import HttpResponse
def home_page_view(request):
return HttpResponse("Hello World!")
And we register the route at the application level:
comments\urls.py
from .views import home_page_view
urlpatterns = [
path("", home_page_view, name="home"),
]
And we register the route at the project level:
mystore\urls.py
from django.contrib import admin
from django.urls import path, include # new
urlpatterns = [
path("admin/", admin.site.urls),
path("", include("comments.urls")), # new
]
And also the application:
mystore\settings.py
INSTALLED_APPS = [
***
"comments", # new
]
We will evaluate the details of what we did in the following sections.
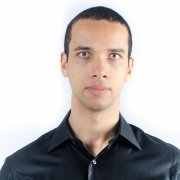
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter