What are Adapters and how do you configure them to display Lists and Grids in Android? (Part 1)
- Andrés Cruz
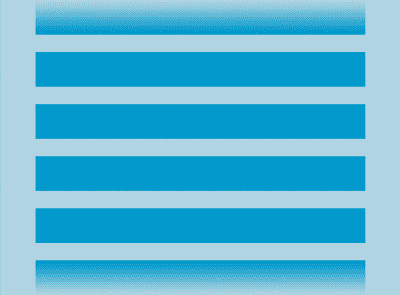
We can say that the main objective of most of the applications developed for mobile devices, including Android, is to consume content: news, notes or other lists of information are some examples; the so-called Adapters are a powerful tool provided in the android.widget API that we can use for this task; it consists of displaying a set of information through lists or grids (customizable at the level of definition, style and events); In this installment we will see how to create a custom Adapter for Android.
What is an Adapter according to the Android API?
In the Android API they define the adapters as:
An Adapter object acts as a bridge between an AdapterView and the underlying data for that view. The Adapter provides access to the data items. The Adapter is also responsible for making a View for each item in the data set.
A possible translation of the above would be as follows: An Adapter object acts as a bridge between the AdapterView and the data of a view. The Adapter provides access to each item. The adapter is also responsible for making a view for each product in the data set.
We have several elements here:
- Data Collection: Data to be displayed; they are generally stored in a List, ArrayList or array.
- View: Definition of how an element of the list will look through an XML layout.
- Adapter: Finally we define the bridge between the data and the view; the Adapter is in charge of taking the view and repeating it for each data collection as well as interacting with the elements that make up the view.
Basically we can use an interface of an Adapter to create and display lists of information from a list of objects or other data types in an efficient way and interact with it through events.
Defining an Adapter in four steps...
In order to work with the Adapters that we will see in this installment, we have to perform the following steps:
- Define the style of our list through a layout (XML).
- Create a class (Adapter) that extends from ArrayAdapter<?>, where ? It can be an object or a data type.
- Add a Tag or ListView or GridView element as the case may be to the layout of the activity in which we want to include the list; If we want our list to be in the form of a list:
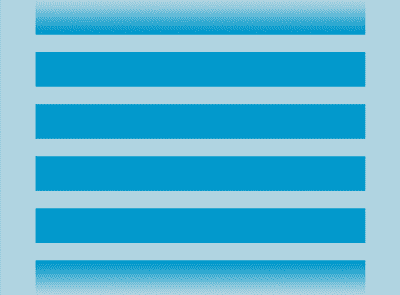
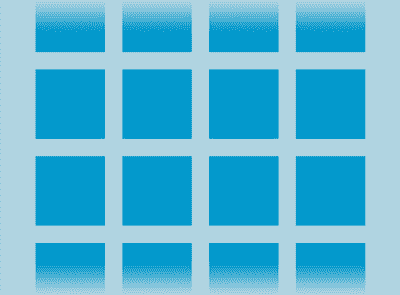
- We must use GridView.
- Invoke the Adapter from an Activity.
1. Creating the look of our list
We define the layout of the Adapter inside the layout folder that looks like this:
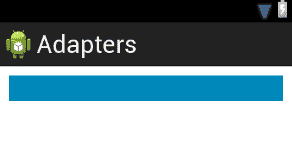
<?xml version = "1.0" encoding = "utf-8"
?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:layout_margin = "10dp"
android:background = "#0088BB"
android:orientation = "vertical"
android:padding = "5dp" >
<TextView
android:id = "@+id/nameTextView"
android:layout_width = "fill_parent"
android:layout_height = "wrap_content"
android:textColor = "#FFFFFF" />
</LinearLayout/>
This layout represents the appearance that each item in the list will have, as you can see, it only represents the appearance of an item in the data collection, it is up to the Adapter to repeat the process for each of the elements that make up the collection of data. data.
This layout can be as complex as you like; It can count on several text fields (TextView), buttons (Button) or any other elements offered by the Android API.
2. Creating the ArrayAdapter
Once the layout is defined and created, the next step is to create a class that extends ArrayAdapter<String> with the following content:
public class ListAdapter extends ArrayAdapter<String> {
private Activity activity;
ArrayList<String> mensajes;
public ListAdapter(Activity activity, ArrayList<String> mensajes) {
super(activity, R.layout.list_view);
this.activity = activity;
this.mensajes = mensajes;
}
static class ViewHolder {
}
public int getCount() {
return mensajes.size();
}
public long getItemId(int position) {
return position;
}
public View getView(final int position, View convertView,
final ViewGroup parent) {
View view = NULL;
return view;
}
}
ViewHolder: Allows you to reference the elements that make up the list; In our example, the list is only made up of a TextView:
static class ViewHolder {
protected TextView nameTextView;
}
And the getView; where the values of the view elements are referenced and set:
// inflamos nuestra vista con el layout
View view = NULL;
LayoutInflater inflator = activity.getLayoutInflater();
view = inflator.inflate(R.layout.list_view, NULL);
final ViewHolder viewHolder = new ViewHolder();
// *** instanciamos a los recursos
viewHolder.nameTextView = (TextView) view
.findViewById(R.id.nameTextView);
// importante!!! establecemos el mensaje
viewHolder.nameTextView.setText(mensajes.get(position));
return view;
- We inflate the menu with our layout already defined in step one.
- We reference the elements of the layout (the TextView).
- And we do something with the already referenced elements, in this example we set a value from the messages list.
An interesting point is that it is possible to define the events inside the Adapter as we will see in the next article.
3. Adding the Adapter to the layout of the Activity (Activity)
We define the following element within the main_layout.xml or the layout of the activity:
<ListView
android:id="@+id/listview"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
4.Calling the Adapter from the Activity
Now all that remains is to call the Adapter from the Activity:
// creamos nuestra coleccion de datos
mensajes = new ArrayList<String>();
mensajes.add("uno");
mensajes.add("dos");
mensajes.add("tres");
mensajes.add("cuatro");
mensajes.add("cinco");
// creamos el listado
listAdapter = new ListAdapter(this, mensajes);
// establecemos el adaptador en la lista
listView.setAdapter(listAdapter);
When running the application, we will see a screen like this:
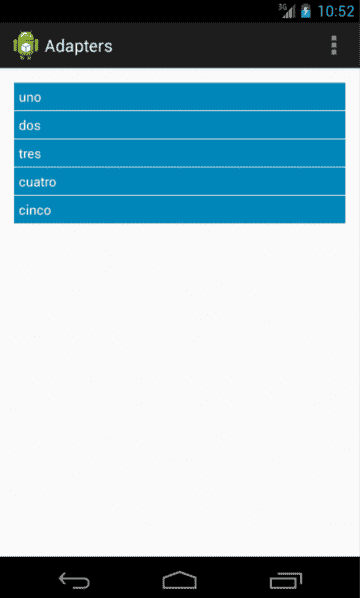
You can see the complete code of the example in our repository GitHub.
I agree to receive announcements of interest about this Blog.
The Adapters consist of displaying a set of information through lists or grids (customizable at the level of definition, style and events).
- Andrés Cruz