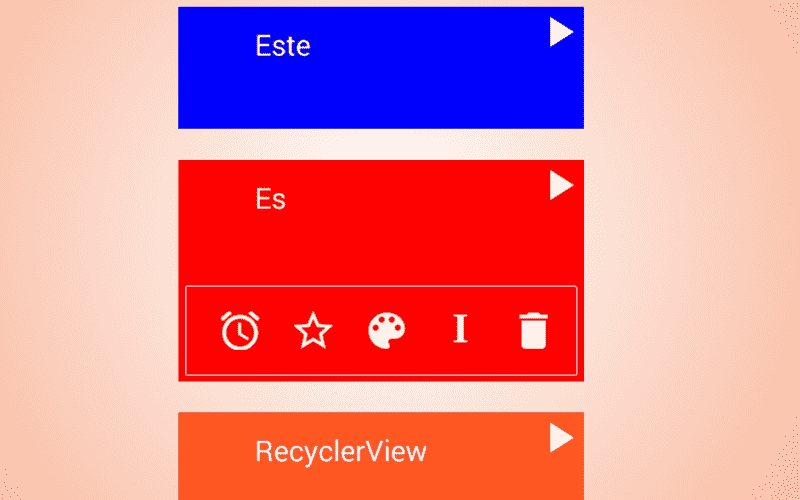
The RecyclerView, like the GridView and ListView, allow you to create lists of elements either through lists or cells; the RecyclerView can be seen as a more flexible, powerful and updated version than these and surely at some point they will be their definitive replacement; the way it works is the same used as its predecessors as you can see in this image:

Adapter that acts as a bridge between the data and the view.
Add the support library to work with the RecyclerView and CardView
In the same way that we added the necessary dependencies to add multiple elements of the Support Library (specifically the FloatActionButton), it is necessary to add the dependencies to be able to use the RecyclerView and CardView in our project; add the following dependencies in the build.gradle file:
compile 'com.android.support:cardview-v7:23.0.1'
compile 'com.android.support:recyclerview-v7:23.0.1'
app/build.gradle
The CardView
The RecyclerView and the CardView are part of the support library; The CardView inherits from the ViewGroups more directly from the FrameLayout and therefore it is an element that allows us to define many other elements within it, such as the following:
<android.support.v7.widget.CardView xmlns:card_view="http://schemas.android.com/apk/res-auto"
android:id="@+id/card_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_margin="2dp"
card_view:cardCornerRadius="1dp">
<RelativeLayout
android:id="@+id/parent_body_rl"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#FF5722"
android:orientation="vertical"
android:padding="2dp">
<LinearLayout
android:id="@+id/parent_body_ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="2dp">
<LinearLayout
android:id="@+id/color_ll"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center"
android:layout_margin="10dp"
android:background="#FF0000"
android:orientation="vertical" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical"
android:padding="2dp">
<TextView
android:id="@+id/name_tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:text="texto 1"
android:textColor="#FFFFFF"
android:textSize="25sp" />
<TextView
android:id="@+id/description_tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/info_text"
android:padding="10dp"
android:text="texto 2"
android:textColor="#FFFFFF"
android:textSize="15sp" />
</LinearLayout>
</LinearLayout>
</RelativeLayout>
</android.support.v7.widget.CardView>
Obtaining the following result:

In other words, we can use the CardView in conjunction with the RecyclerView; where the CardView defines the items of the list.
Creating a RecyclerView
First of all, you need to add a RecyclerView element in the layout of our Activity or Fragment:
<android.support.v7.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/recycler_view"
/>
Like any other element, to reference the previous RecyclerView from our Activity we use the following Java code:
RecyclerView recyclerView = (RecyclerView)findViewById(R.id.recycler_view);
Specifying the positioning of the RecyclerView with the Layout Manager
From here we can appreciate some changes with respect to the moment of creating the ListView and GridView; to define the position in which we want our list of items to render (in the form of lists or cells):
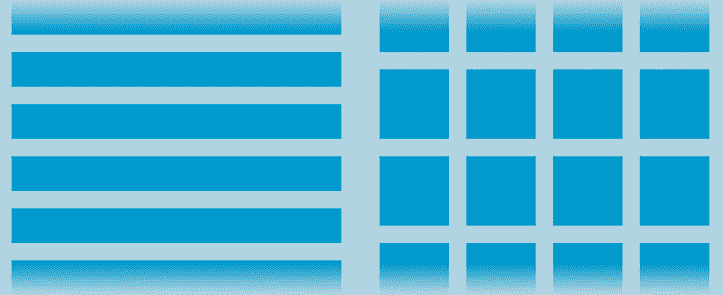
We use the following Java code so that the elements are positioned in the list through listings:
LinearLayoutManager linearLayoutManager = new LinearLayoutManager(context); recyclerView.setLayoutManager(LinearLayoutManager);
Or the GridLayoutManager so that the elements are positioned in the list via cells:
GridLayoutManager gridLayoutManager = new GridLayoutManager(context); recyclerView.setLayoutManager(gridLayoutManager);
Specifying the data and the model
For greater ease when manipulating the data, we will create a Person model to specify a list with our data that we will later pass to the Adapter.
public class Person {
String name;
String description;
String color;
Person(String name, String description,String color){
this.name = name;
this.description = description;
this.color = color;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
}
Nothing out of the ordinary, the person has a name, a description (age, etc.) and the favorite color.
Defining the Adapter
The Adapter used by RecyclerViews are very similar to those used by ListViews and GridViews in terms of their structure and behavior; in addition to this, we are going to use the ViewHolder to more easily reference the elements that interest us in our list; the definition of the Adapter below:
public class ListAdapter extends RecyclerView.Adapter<ListAdapter.ViewHolder>{
private ArrayList<Person> persons;
// Provee una referencia a cada item dentro de una vista y acceder a ellos facilmente
public static class ViewHolder extends RecyclerView.ViewHolder {
// Cada uno de los elementos de mi vista
public TextView nameTextView,descriptionTextView;
public CardView cardView;
public LinearLayout colorLl;
public RelativeLayout parentBodyRl;
public ViewHolder(View v) {
super(v);
parentBodyRl = (RelativeLayout) v.findViewById(R.id.parent_body_rl);
cardView = (CardView) v.findViewById(R.id.card_view);
nameTextView = (TextView) v.findViewById(R.id.name_tv);
descriptionTextView = (TextView) v.findViewById(R.id.description_tv);
colorLl = (LinearLayout) v.findViewById(R.id.color_ll);
}
}
// Constructor
public ListAdapter(ArrayList<Person> persons) {
this.persons = persons;
}
// Create new views (invoked by the layout manager)
@Override
public ListAdapter.ViewHolder onCreateViewHolder(ViewGroup parent,
int viewType) {
// inflo la vista (vista padre)
View v = LayoutInflater.from(parent.getContext()).inflate(R.layout.list_adapter, parent, false);
// creo el grupo de vistas
ViewHolder vh = new ViewHolder(v);
return vh;
}
// Reemplaza en contenido de la vista
@Override
public void onBindViewHolder(ViewHolder viewHolder, final int position) {
viewHolder.nameTextView.setText(persons.get(position).getName());
viewHolder.descriptionTextView.setText(persons.get(position).getDescription());
}
// Retorna el tamano de nuestra data
@Override
public int getItemCount() {
return persons.size();
}
}
As we see in the previous code, there are three methods that we must override:
The getItemCount() method that should return the size of the list, or what is the same, the size of our list of items that will be displayed in the list.
The onCreateViewHolder() method allows us to initialize all the elements that make up our previously defined ViewHolder class; we also define the layout (which can vary for each element) that each element uses. It is also possible to define the events in this function.
In the onBindViewHolder() method, the values of the different fields must be defined or bound.
Invoking the above Adapter
From our Activity or Fragment, we create an object of the Adapter defined above:
ListAdapter listAdapter = new ListAdapter(persons); audioRv.setAdapter(listAdapter);
And when running our application on the emulator or physical device:
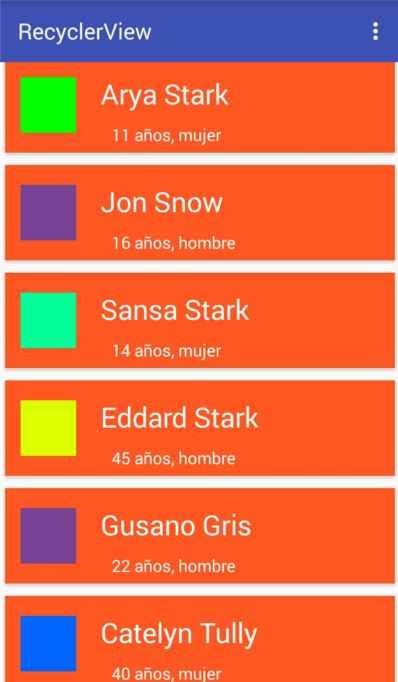
Conclusions
In this post we took the first steps with the RecyclerView and CardView available in the latest versions of Android and later through the Support Library; we could notice that the operation and the implementation are very similar to what we are used to with the GridView and ListView; In the following posts we will see how easy it is to implement other functionalities to the RecyclerView and CardView, such as the swipe and some simple animations to hide sections of the CardView.
I agree to receive announcements of interest about this Blog.
The RecyclerView, like the GridView and ListView, allow you to create lists of items either through lists or cells and are a more flexible, powerful and updated version than the GridView ListView.
- Andrés Cruz