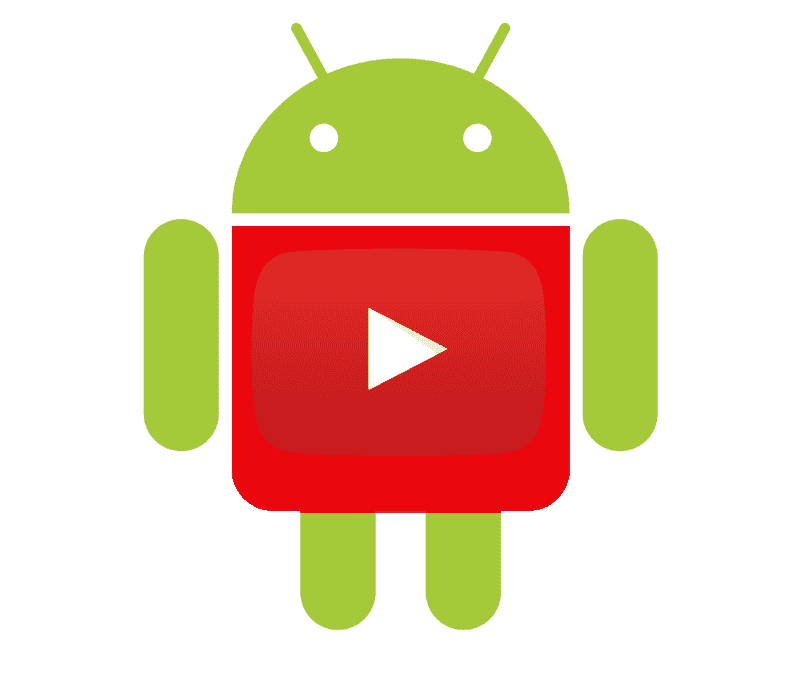
After many days without being able to publish an entry due to excessive work that I have had these days, today we will see how to embed a YouTube video in an Android application using the native YouTube API created by Google.
As it has become regular, we will use Android Studio instead of Eclipse with the ADT plugin.
The experiment is simple, although before being able to embed a video we must carry out a series of steps.
Android Manifest
The first thing we must do is place the corresponding permissions in our Android Manifest and indicate that our application requires an Internet connection:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
Getting access to the Youtube API via a jar
Now we must download a jar file that will give us access to the YouTube API; Said jar is currently in version 1.2.2 and we can obtain it from the following link: YouTube Android Player
API - Download.
Once the previous file has been downloaded, we unzip it and locate ourselves in the libs folder and there is our jar.
Copying the jar into our project
As you can see, this time we do not use a dependency via gradle as it would be normal, we will use the previously obtained jar that we located in the lib folder of our project:
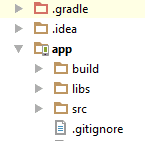
Adding dependency via jar in the gradle
Now we must edit our gradle to tell it where our jar is:
compile fileTree(include: ['*.jar'], dir: 'libs')
It is important to note that there is an API to access via gradle in the YouTube Data API Client Library for Java, although it offers other features than those of the previous jar.
Remember to synchronize the gradle and be aware if it throws any errors.
With our project already configured, now we can use the Youtube API to place a video; well, the truth is that there is a crucial step missing.
Creating our project in the Google Developer Console
As it has been normal when we want to use any Google API in an Android application or another platform such as the web, we must carry out a series of configurations to have access to the API in question; We achieve this by logging in with our account through the Google developer console.
The first thing we need to do is create a project:
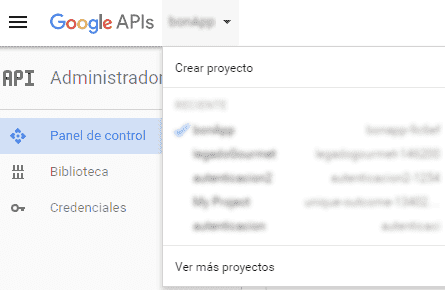
We enable the Youtube API:
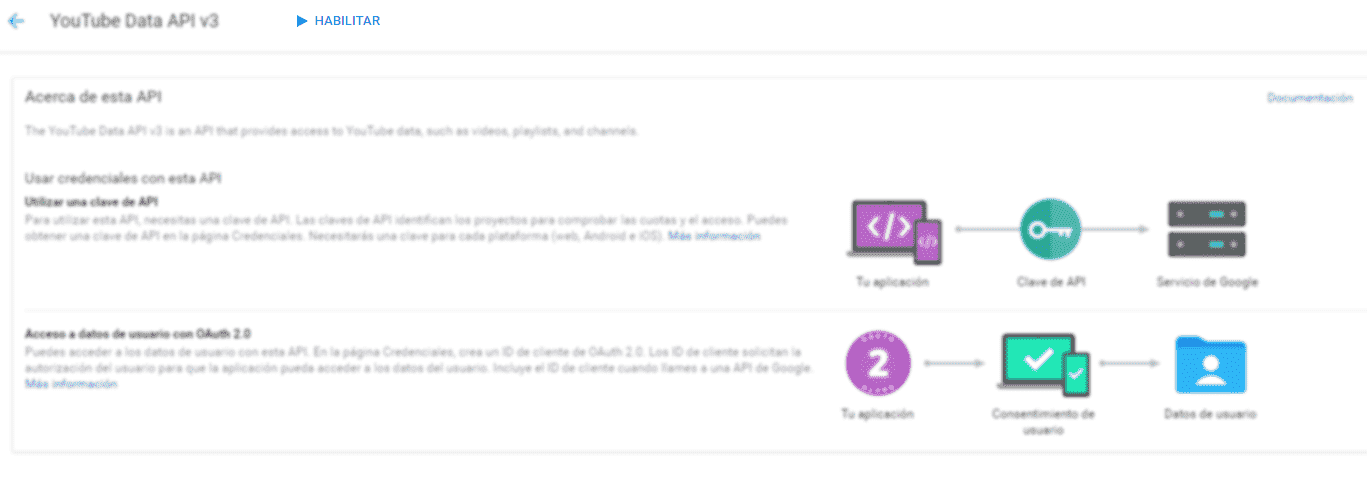
Then we click on "Credentials" => "Create credentials" => "API Key":
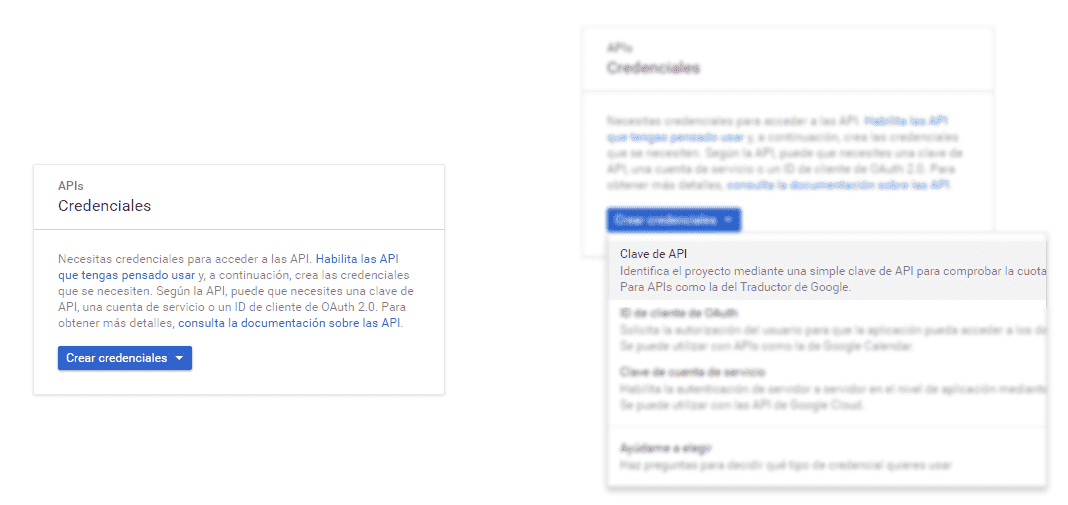
We will be presented with a form like the following:
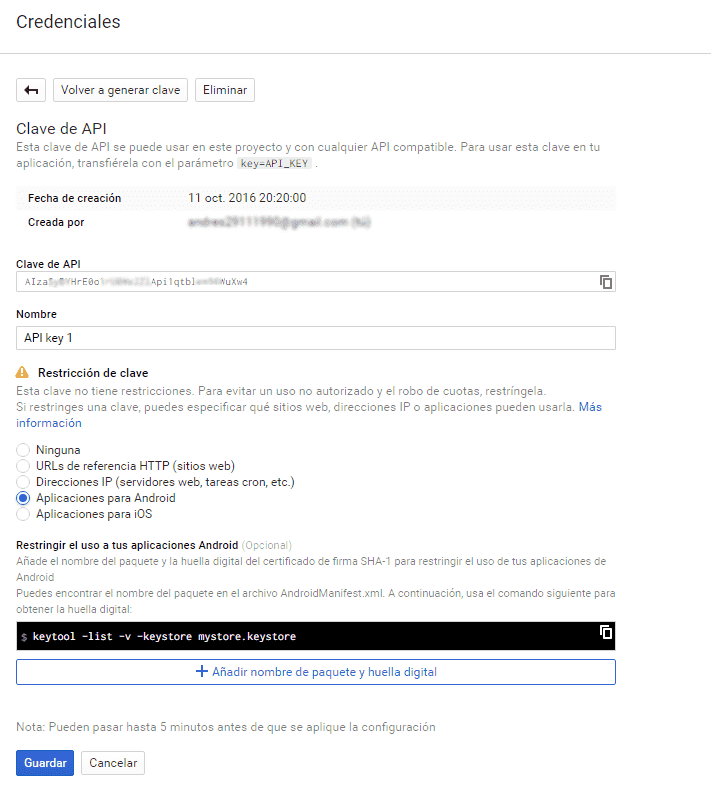
Where we must add the fingerprint of our application; To achieve it we can use the following command (once located inside the bin folder of the java SDK in case of not having configured the environment variables):
keytool -list -v -keystore "%USERPROFILE%\.android\debug.keystore" -alias androiddebugkey -storepass android -keypass android
With this we get:

And we enter it with the name of our package in the previous form.
Now we copy the key of our project from the Google developer console:

We will create an extra file where we will place the key obtained in the Google developer console that will have the following content:
public class ConfigYoutube {
// Google Console APIs developer clave
public static final String DEVELOPER_KEY = "clave-consola-desarrolladores-google";
// YouTube video id
public static final String YOUTUBE_VIDEO_CODE = "_oEA18Y8gM0";
}
And now we can use the YouTube API in our Android application! (this time if it's true).
Layout
We create a Fragment element in the layout of our activity:
<fragment
android:id="@+id/youtubeplayerfragment"
android:name="com.google.android.youtube.player.YouTubePlayerFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_weight="8" />
We will create an activity like the following:
public class VideoActivity extends YouTubeBaseActivity
implements YouTubePlayer.OnInitializedListener {
public static final String DEVELOPER_KEY = ConfigYoutube.DEVELOPER_KEY;
private static String VIDEO_ID = ConfigYoutube.YOUTUBE_VIDEO_CODE;
private static final int RECOVERY_DIALOG_REQUEST = 1;
YouTubePlayerFragment myYouTubePlayerFragment;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_video);
myYouTubePlayerFragment = (YouTubePlayerFragment) getFragmentManager()
.findFragmentById(R.id.youtubeplayerfragment);
myYouTubePlayerFragment.initialize(ConfigYoutube.DEVELOPER_KEY, VideoActivity.this);
}
@Override
public void onInitializationFailure(Provider provider,
YouTubeInitializationResult errorReason) {
if (errorReason.isUserRecoverableError()) {
errorReason.getErrorDialog(this, RECOVERY_DIALOG_REQUEST).show();
} else {
String errorMessage = String.format(
"There was an error initializing the YouTubePlayer (%1$s)",
errorReason.toString());
Toast.makeText(this, errorMessage, Toast.LENGTH_SHORT).show();
}
}
@Override
public void onInitializationSuccess(Provider provider, YouTubePlayer player,
boolean wasRestored) {
if (!wasRestored) {
player.cueVideo(VIDEO_ID);
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == RECOVERY_DIALOG_REQUEST) {
// Retry initialization if user performed a recovery action
getYouTubePlayerProvider().initialize(DEVELOPER_KEY, this);
}
}
protected Provider getYouTubePlayerProvider() {
return (YouTubePlayerView) findViewById(R.id.youtubeplayerfragment);
}
}
As you can see, it is nothing more than an activity that extends YouTubeBaseActivity, we overwrite some methods and with this we are able to embed our YouTube video.
I agree to receive announcements of interest about this Blog.
It explains how to embed a Youtube video in an Android application using the native Youtube API created by Google.
- Andrés Cruz