Among the resources that are part of the huge Android API there is one that allows you to display web pages through a class called WebView; with the help of the WebView class we can create our own browser and how can you imagine using the WebKit engine.
Is the WebView class a browser?
WebView is not a browser since it does not have elements present in browsers such as the address bar or other navigation controls; it only allows you to display a web page in a native Android application.
Although with the WebView class we can decide if we want to display the content within the application or in a web browser as we will see later.
Building Web Apps with WebView
To add a WebView in our Android application we must carry out a series of configurations that we will see below:
Enable Internet permission in the AndroidManifest
One of the things that we must first do is request the necessary permission in our AndroidManifest so that the application can connect to the Internet and be able to download the HTML content:
<manifest ... >
<uses-permission android:name="android.permission.INTERNET" />
...
</manifest>
Adding the WebView tag in the layout
Once the permission is requested, we add the WebView tag in the layout of the Android activity:
<WebView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/webview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
Loading the web page in the WebView
Finally we can add the URL of the web page that we want to display through the method loadUrl()
:
WebView webView = (WebView) this.findViewById(R.id.webview);
webView.loadUrl("");
In our application we will add the following URL referenced in the code:
webView.loadUrl("http://desarrollolibre.net/blog/tema/150/javascript/como-hacer-una-sencilla-galeria-con-css-y-6-lineas-de-javascript");
Managing page navigation
Now at this point we can run our Android application; but when executing it we will see a screen like the following:
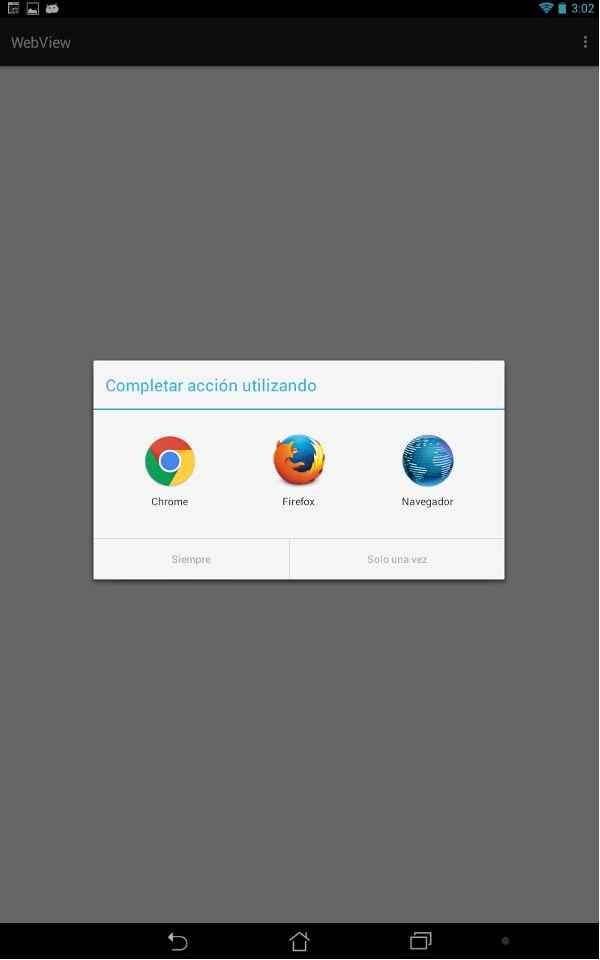
The idea of creating a Web App with the WebView class is not that we simply open the content in one of the web browsers that we have installed on the Android device, but that it runs inside our application; for this we will see the following block.
The WebViewClient class
As we discussed above; by default Android launches an application (like web browsers) that handles the previously set URL; but we can customize this behavior to be handled internally by our application; in other words, have the web page run directly in our application.
We simply create an instance of the WebViewClient class and it is the minimum we need to display a web page within our application:
webView.setWebViewClient(new WebViewClient());
But if we want more control we can create our own class that extends WebViewClient and override certain methods:
private class MyWebViewClient extends WebViewClient { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { return true; } }
You can find the complete information in the link.
Enabling the JavaScript
JavaScript is disabled by default in the WebView, which can be a problem depending on the type of web page you want to load; that is, if our page to be loaded through the loadUrl() method needs JavaScript; we can easily enable the Internet through the setJavaScriptEnabled() method; Let's see how to use it:
First we create a WebSettings object that allows us to make various configurations, although in our case, we are simply interested in enabling JavaScript:
WebSettings webSettings = myWebView.getSettings();
Now we can use the setJavaScriptEnabled() method passing the boolean true as a parameter.
webSettings.setJavaScriptEnabled(true);
The WebView class in practice
Finally let's see the code of our application:
The layout
<WebView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/webview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
The Java code
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
WebView webView = (WebView) this.findViewById(R.id.webview);
webView.loadUrl("http://desarrollolibre.net/blog/tema/150/javascript/como-hacer-una-sencilla-galeria-con-css-y-6-lineas-de-javascript");
webView.setWebViewClient(new WebViewClient());
}
When running the code:
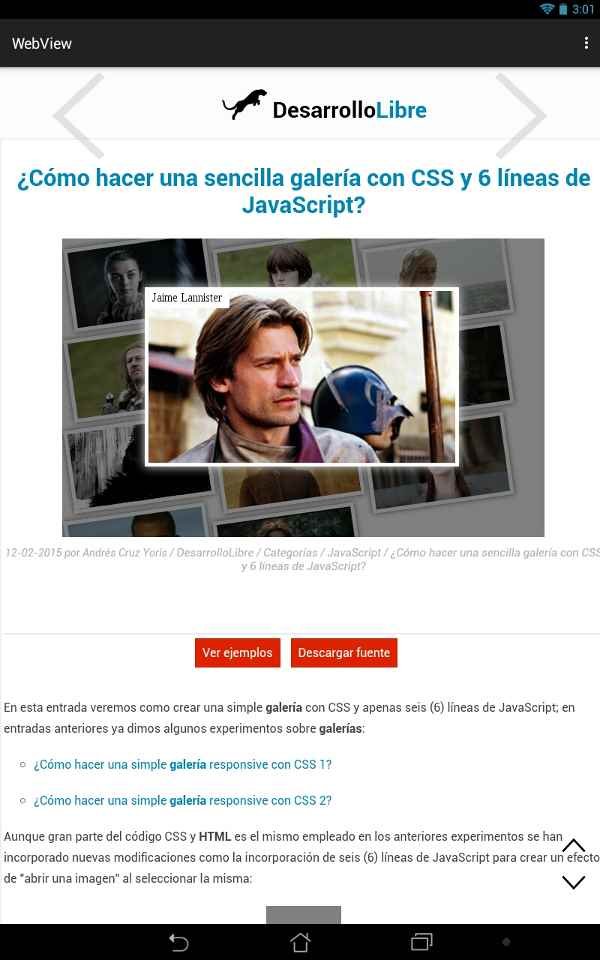
You can find the complete code of the Android application in our GIT repository:
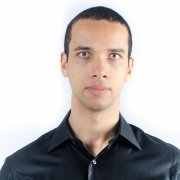
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter