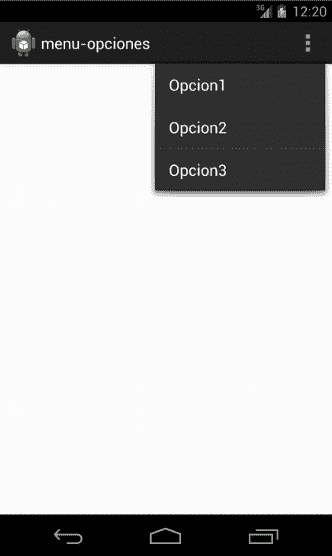
Menus are key User Interface components for any system; in Android, the menus present options that allow you to perform actions such as moving between the different activities that make up an Android application.
Drop down a menu in Android
The Android applications menu is displayed by pressing the icon corresponding to the menu, generally located in the upper right corner of the screen:
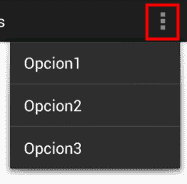
In this post we will see how to create a basic options menu for Android
Ways to create a menu in Android
There are two ways to create an options menu in Android, through an XML of items, which is what we will see in this entry, and through code (recommended when you want to perform a specific task with the menu); the IDE to use: Eclipse with the ADT plugin.
Define an XML-based menu
For all types of menus, Android provides an XML format to define menu elements instead of building a menu with code in an activity; Creating a menu in this way has the main advantage that it is easier to visualize its structure and avoids creating visual elements from the view as the definition of the menu is separated in a separate file from the main code of the application.
XML menu items
- <menu>: It is the container of the elements of a menu; we can say that it is the one that defines a menu; therefore it must be the root node and can contain one or more of the following elements:
- <item>: Represents a menu item; can contain a menu item to make a sub-menu.
- <group>: (optional) It simply allows us to categorize our menu items (<item>); therefore it can only contain <item> inside it.
Menu item attributes (<item>)
- <android:id>: As any element; is an identifier for a unique resource within our menu; which allows the application to recognize it when the user clicks on it.
- <android:title>: The title of the menu; and it will be the text that is reflected in the menu.
- <android:icon>: Add an icon to the element; the icons used must be in the folders: res\drawable
- <android:showAsAction>: Specifies when and how the menu item (<item>) should appear.
Example of a basic menu in Android
Once seen how a menu is formed; we can create a basic example menu; which will consist of three options:
- Option 1
- Option 2
- Option 3
And it will look like this:
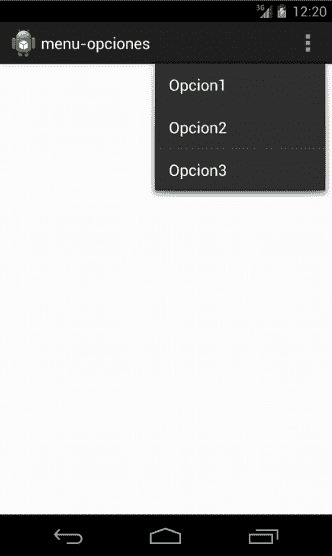
Creating the options menu in Android
To create the previous menu by means of its definition in XML we must create an XML that we will call "menu.xml" located in the directory:
res\menu
And we add the following content:
<?xml version="1.0" encoding="utf-8"?>
<menu
xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/opcion1" android:title="Opcion1"></item>
<item android:id="@+id/opcion2" android:title="Opcion2"></item>
<item android:id="@+id/opcion3" android:title="Opcion3"></item>
</menu>
The menu <menu> is made up of <item>; each with a unique android:id and an android:title.
Finally we must override the onCreateOptionsMenu and onOptionsItemSelected methods in our Activity:
The onCreateOptionsMenu method
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu, menu);
return true;
}
The onCreateOptionsMenu method within the activity that implements the menu allows you to inflate (convert an XML resource, in our case the menu into an object, in this case a Menu) the XML that defines the menu using R.menu.menu that corresponds to the XML that we defined in the previous steps and that is located in the res/menu directory; we return true to display the menu.
The onMenuItemSelected method
@Override
public boolean onMenuItemSelected(int featureId, MenuItem item) {
switch(item.getItemId()) {
case R.id.opcion1:
return true;
case R.id.opcion3:
return true;
case R.id.opcion2:
return true;
}
return super.onMenuItemSelected(featureId, item);
}
Now we override the onMenuItemSelected method to check which of the MenuItems (<item>) we have created has been clicked; the Menuitem parameter has reference to the element that has been clicked by the user; getting the id by calling the getItemId method; through the switch we verify which resource the user has selected to perform a specific task.
This is all we need to create a menu; you can find the complete example in our Android github repository/menu-options or by clicking here.
I agree to receive announcements of interest about this Blog.
These menus appear by pressing the menu option on our device; In this article we will see how to create a basic options menu in XML for our application, how a menu is formed, among others; using eclipse and the ADT plugin.
- Andrés Cruz