A Canvas is nothing more than a surface or canvas on which you can draw; With the help of the primitives offered by the Canvas API in Android, it is possible to draw graphics such as lines, circles, ovals, squares, rectangles and even texts, as well as vary the color, layout and size; In this post we will take the first steps with the Canvas class in Android.
Getting started with Canvas in Android -the onDraw() method-
First you have to define a class that extends View which can be contained within the same activity or outside it; We will call the class MyView that overrides the onDraw(Canvas) method:
public class MyView extends View {
public MyView(Context context) {
super(context);
}
@Override
protected void onDraw(Canvas canvas) {
}
}
The onDraw() method
The onDraw(Canvas canvas) method specifies a Canvas parameter whose class is the one that allows drawing the primitives: lines, circles, rectangles, etc.
Drawing a figure:
To draw a simple figure, we must specify a series of methods together with their parameters to change the style of the paths, colors, shapes, etc:
- Define a paint to specify parameters such as color, line thickness, etc; Let's see some of them:
- paint.setColor: Sets a color to paint; the method receives as a parameter of type color; for example: Color.WHITE
- Paint.setStyle: Sets a style for painting; the method receives a parameter of type Paint.Style which can be:
- FILL: Draw a filled figure:
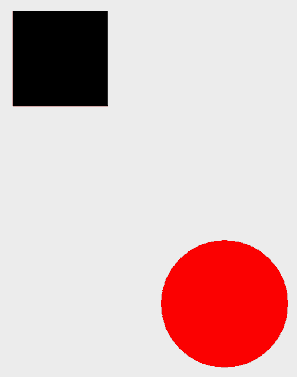
- STROKE: Draw only the outline of the figure:
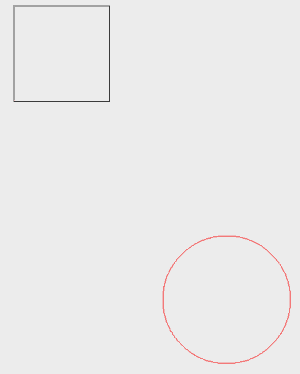
- FILL_AND_STROKE: Combination of the previous two.
- The Canvas defines the colors and geometric shapes to be drawn:
Canvas.drawColor()
: Define a color.Canvas.drawCircle(X,Y,Radius,Paint)
: Draws a circle according to: The specified position (X and Y) radius (Radius) and Paint.Canvas.drawLine(startX,startY,stopX,stopY,Paint)
: Draws a line according to the specified segment (startX,startY,stopX,stopY) and Paint.Canvas.drawRect(left, top, right, bottom, paint))
: Draws a rectangle based on the specified position (left,top,right,bottom) and Paint.Canvas.drawPaint(Paint)
: Paints the entire Canvas according to the specified Paint.
Associating the Canvas in the activity
Once we have defined the view which is going to draw on the Canvas; The next step is to attach an instance of the MyView class to the activity using the setContentView() method:
setContentView(new MyView(this));
When we want to draw graphics in Android we can do it inside the view using a layout (the traditional way of referencing a layout in Android):
setContentView(R.layout.activity_main);
Or draw the graphics directly on a Canvas as we have done in this post:
setContentView(new MyView(this));
As you can see, for both steps we use the setContentView() method but with very different results.
Draw graphics inside the layout or on the Canvas?
It is recommended to draw the graphics in layout when you are going to handle simple graphics without much movement; but, when you want to create richer graphics with more animations, motions, drawing refresh, and better performance, Android recommends using Canvas instead.
You can find a working application that exemplifies the behavior of the codes seen above in our Git repository:
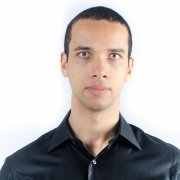
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter