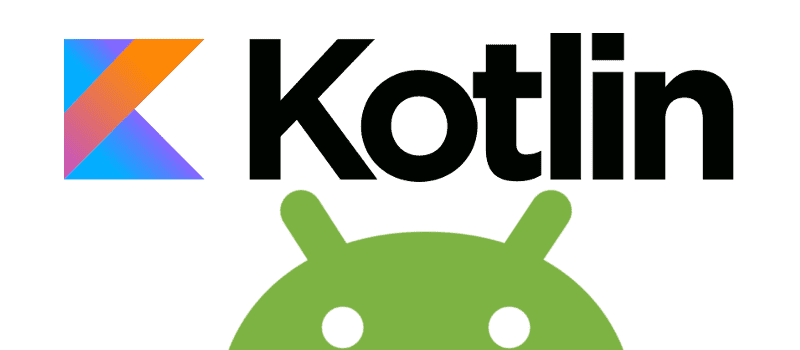
Continuing with the Kotlin tutorials, today we will see the Pair data class in Kotlin, which is simply a generic representation (any type of data or classes) of two values (pairs); they are a kind of tuple but with some variants.
The Pair data class is a structure that allows two values to be stored.
Even values are great when we want to store pairs of data; and that simple, it is a structure that serves us only for that; the constructor method or rather the basic structure of an even value in Kotlin looks like the following:
Pair(first: A, second: B)
The first value corresponds to the first value and the second value, as you can imagine.
Already in practice, to use even values in Kotlin is as easy as doing the following:
var pair = Pair("Kotlin Pair",2)
As you can see, we are not forced to have even values of the same type, they are independent and can take any value we need.
Pair or to keyword to create even values
We can also create even values using the reserved variable to as follows:
var pair = "Kotlin Pair" to 2
This is very useful in Android since we can use the Pair to store a pair of values, for example a username and password or any pair of values that have a relationship.
How to access Pair values in Kotlin?
Having clear what the structure of the Pair in Kotlin is for, the next thing we are interested in knowing is how to access each of these embedded values within a Pair data class; for this, the reserved words first and second are used respectively in the following way; having our variable named pair defined in any of the above ways:
println(pair.first) //Kotlin
println(pair.second) //Pair2
And we get as output:
Kotlin
Pair2
We can also use the component1() and component2() methods respectively to get the same output as above:
println(pair.component1()) //Kotlin
println(pair.component2()) //Pair2
For each of the println respectively.
Decomposition of Pair values in Kotlin
We can also separate or decompose the values of the pairs into individual and independent variables in the following way; as we did before with the same data class in the previous entry:
val (user, password) = Pair("usuario", "contrasena")
println(user) // usuario
println(password) // contrasena
And you get:
usuario
contrasena
Always remember to review the official documentation at: Kotlin: Pair and Kotlin: To.
Even Value Methods
Pairs in Kotlin have a couple of methods that we can use to use the entire structure 100%; the first of them is the toString that prints a string with the values two values:
var pair = Pair("Kotlin Pair",2)
fun main(args: Array<String>) {
println(pair.toString()) // imprime (Kotlin Pair, 2)
}
And we also have the toList() method that returns a list with both even values:
var pair = Pair("Kotlin Pair",2)
fun main(args: Array<String>) {
println(pair.toList()) // imprime [Kotlin Pair, 2]
}
Inspiration to use even values
With the even values we can do many things, there are many structures that are perfect for us to use a Kotlin Pair; for example the location of a pair of points on a 2D plane:
data class Punto<T>(var x: T, var y: T)
Which can be serializable so you can put in any value, such as integers or floats; we can use it as a pair like we did earlier for a username and password pair, we can also use it to set up a URL and a single parameter:
var url = Pair("https://twitter.com/","ACY291190")
I agree to receive announcements of interest about this Blog.
Data classes in Kotlin allow us to store data and only data through a simple structure similar to that of a class; we can print hashes, clone objects, and print values from generic methods and much more.
- Andrés Cruz